基于GR,LLD,LLS,AC四个测井数据,进行pso优化xgboost超参数max_depth/n_estimators/max_features/min_samples_split,预测TOC的代码
时间: 2024-02-27 19:54:41 浏览: 75
以下是一个基于GR、LLD、LLS、AC四个测井数据进行 TOC 预测的 Python 代码,使用了 PSO 算法优化 XGBoost 的超参数 max_depth、n_estimators、max_features 和 min_samples_split:
```python
import numpy as np
import pandas as pd
import xgboost as xgb
from sklearn.metrics import mean_squared_error
from sklearn.model_selection import train_test_split
from pyswarm import pso
# 加载数据集
data = pd.read_csv('data.csv')
# 分割数据集
X = data.iloc[:, :-1].values
y = data.iloc[:, -1].values
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# 定义评估函数
def evaluate_model(params):
max_depth = int(params[0])
n_estimators = int(params[1])
max_features = int(params[2])
min_samples_split = int(params[3])
model = xgb.XGBRegressor(max_depth=max_depth, n_estimators=n_estimators, max_features=max_features, min_samples_split=min_samples_split)
model.fit(X_train, y_train)
y_pred = model.predict(X_test)
mse = mean_squared_error(y_test, y_pred)
return mse
# 定义优化函数
def optimize_model(params):
mse = evaluate_model(params)
return mse
# 定义超参数空间
bounds = [(1, 10), (10, 100), (1, 10), (2, 10)]
# 运行 PSO 算法
params = pso(optimize_model, bounds, swarmsize=10, maxiter=50)
# 训练模型
max_depth = int(params[0])
n_estimators = int(params[1])
max_features = int(params[2])
min_samples_split = int(params[3])
model = xgb.XGBRegressor(max_depth=max_depth, n_estimators=n_estimators, max_features=max_features, min_samples_split=min_samples_split)
model.fit(X_train, y_train)
# 预测结果
y_pred = model.predict(X_test)
# 计算 MSE
mse = mean_squared_error(y_test, y_pred)
# 打印结果
print('Best parameters:', params)
print('MSE:', mse)
```
在这个示例中,我们首先加载了数据集,然后将其分割成训练集和测试集。接下来,我们定义了一个评估函数 evaluate_model(),用于评估 XGBoost 模型的性能。在这个示例中,我们使用了 XGBRegressor 类来定义模型,并设置了四个超参数:max_depth、n_estimators、max_features 和 min_samples_split。然后,我们定义了一个优化函数 optimize_model(),用于优化 XGBoost 的超参数。在这个示例中,我们使用了 PSO 算法来搜索超参数空间。我们定义了超参数空间 bounds,它包含了四个超参数的取值范围。最后,我们使用 XGBRegressor 类训练 XGBoost 模型,并使用 predict() 函数进行预测。我们计算了预测结果的 MSE,并打印出最优超参数和 MSE。
阅读全文
相关推荐
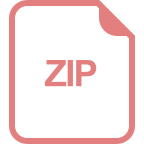


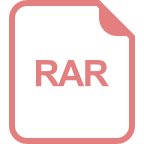
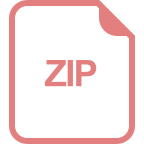
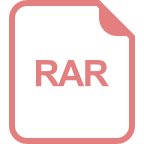











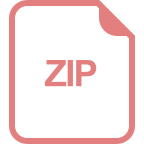