ocr文字识别python
时间: 2024-10-22 21:00:28 浏览: 22
OCR(Optical Character Recognition,光学字符识别)技术是通过计算机软件将图像中的文本转换成可编辑、可搜索的电子形式。在Python中,有许多库可以帮助进行OCR文字识别,其中最常用的是Tesseract和PyTesseract。
1. Tesseract:这是一个开源的OCR引擎,由Google维护。PyTesseract是其Python接口,它允许我们方便地将图片中的文本提取出来。安装通常需要先安装Tesseract本身,然后通过pip安装pytesseract。
```bash
pip install pytesseract Pillow
```
2. PIL (Pillow):它是Python Imaging Library的一个分支,用于处理位图图像,常用于预处理图像以便于OCR。
使用例子:
```python
import pytesseract
from PIL import Image
def ocr_image(image_path):
image = Image.open(image_path)
text = pytesseract.image_to_string(image, lang='chi_sim') # 语言设置可根据需求选择
return text
image_path = "path/to/your/image.jpg"
print(ocr_image(image_path))
```
相关问题
ocr文字识别 python
你可以使用 Python 来实现 OCR(Optical Character Recognition)文字识别。有几个开源库可以帮助你完成这个任务,其中最受欢迎的是 Tesseract。以下是一个使用 Tesseract 进行 OCR 的简单示例:
首先,确保已经安装了 Tesseract。你可以通过以下命令在终端中安装 Tesseract:
```
sudo apt-get install tesseract-ocr
```
然后,你可以使用 `pytesseract` 库来调用 Tesseract 进行文字识别。如果你还没有安装 `pytesseract`,可以通过以下命令安装:
```
pip install pytesseract
```
接下来,你需要准备一张包含文本的图片。假设你的图片路径为 `image.jpg`,你可以使用以下代码来进行文字识别:
```python
import pytesseract
from PIL import Image
# 打开图片
image = Image.open('image.jpg')
# 使用 Tesseract 进行 OCR
text = pytesseract.image_to_string(image, lang='eng')
# 打印识别的文本
print(text)
```
在上述代码中,`image_to_string` 函数将图片转换为文本,并使用 `eng` 作为默认的语言参数。你可以根据需要更改语言参数。
希望这可以帮助到你开始进行 OCR 文字识别的工作!如果有任何问题,请随时问我。
ocr文字识别python代码
以下是一个简单的Python OCR文字识别代码示例:
```
# 导入OCR API
import pytesseract
# 导入图像处理库
from PIL import Image
# 打开图像
image = Image.open('image.png')
# 将图像转换成灰度图像
gray_image = image.convert('L')
# 使用OCR API识别文本
text = pytesseract.image_to_string(gray_image)
# 打印结果
print(text)
```
在运行此代码之前,您需要安装pytesseract和Pillow库。安装方法如下:
```
pip install pytesseract
pip install Pillow
```
请记住,在使用此代码时,请将'image.png'替换为您要识别的图像的文件名。
阅读全文
相关推荐
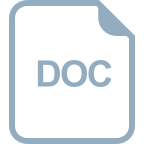
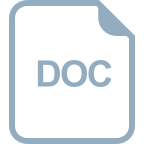
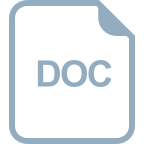
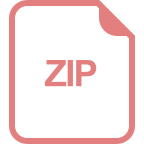
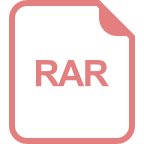
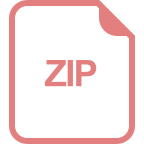
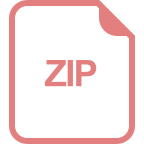






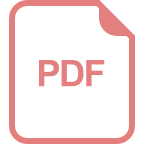
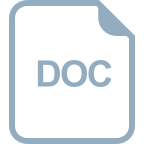
