代码实现 一元稀疏多项式计算器
时间: 2023-11-21 21:54:58 浏览: 79
一元稀疏多项式计算器是一个可以对一元稀疏多项式进行加、减、乘、求导等运算的计算器。其代码实现需要使用数据结构中的链表来存储多项式的系数和指数,具体实现步骤如下:
1. 定义一个结构体来存储多项式的系数和指数,例如:
```
struct PolyNode {
int coef; // 系数
int expon; // 指数
struct PolyNode *next; // 指向下一个节点的指针
};
```
2. 定义一个链表来存储多项式,链表的每个节点都是一个PolyNode结构体,例如:
```
typedef struct PolyNode *Polynomial;
struct PolyNode {
int coef; // 系数
int expon; // 指数
struct PolyNode *next; // 指向下一个节点的指针
};
```
3. 实现多项式的输入函数,可以通过读入系数和指数来构建多项式链表,例如:
```
Polynomial ReadPoly() {
Polynomial P, rear, t;
int c, e;
P = (Polynomial)malloc(sizeof(struct PolyNode));
P->next = NULL;
rear = P;
scanf("%d %d", &c, &e);
while (c != 0) {
t = (Polynomial)malloc(sizeof(struct PolyNode));
t->coef = c;
t->expon = e;
t->next = NULL;
rear->next = t;
rear = t;
scanf("%d %d", &c, &e);
}
return P;
}
```
4. 实现多项式的输出函数,可以遍历多项式链表并输出每个节点的系数和指数,例如:
```
void PrintPoly(Polynomial P) {
int flag = 0;
if (!P) {
printf("0 0\n");
return;
}
while (P) {
if (!flag) {
flag = 1;
} else {
printf(" ");
}
printf("%d %d", P->coef, P->expon);
P = P->next;
}
printf("\n");
}
```
5. 实现多项式的加法函数,可以遍历两个多项式链表并将相同指数的项相加,例如:
```
Polynomial Add(Polynomial P1, Polynomial P2) {
Polynomial front, rear, temp;
int sum;
rear = (Polynomial)malloc(sizeof(struct PolyNode));
front = rear;
while (P1 && P2) {
if (P1->expon > P2->expon) {
temp = (Polynomial)malloc(sizeof(struct PolyNode));
temp->coef = P1->coef;
temp->expon = P1->expon;
temp->next = NULL;
rear->next = temp;
rear = temp;
P1 = P1->next;
} else if (P1->expon < P2->expon) {
temp = (Polynomial)malloc(sizeof(struct PolyNode));
temp->coef = P2->coef;
temp->expon = P2->expon;
temp->next = NULL;
rear->next = temp;
rear = temp;
P2 = P2->next;
} else {
sum = P1->coef + P2->coef;
if (sum) {
temp = (Polynomial)malloc(sizeof(struct PolyNode));
temp->coef = sum;
temp->expon = P1->expon;
temp->next = NULL;
rear->next = temp;
rear = temp;
}
P1 = P1->next;
P2 = P2->next;
}
}
while (P1) {
temp = (Polynomial)malloc(sizeof(struct PolyNode));
temp->coef = P1->coef;
temp->expon = P1->expon;
temp->next = NULL;
rear->next = temp;
rear = temp;
P1 = P1->next;
}
while (P2) {
temp = (Polynomial)malloc(sizeof(struct PolyNode));
temp->coef = P2->coef;
temp->expon = P2->expon;
temp->next = NULL;
rear->next = temp;
rear = temp;
P2 = P2->next;
}
rear->next = NULL;
temp = front;
front = front->next;
free(temp);
return front;
}
```
6. 实现多项式的乘法函数,可以遍历两个多项式链表并将每一项相乘,然后将结果插入到一个新的多项式链表中,例如:
```
Polynomial Mult(Polynomial P1, Polynomial P2) {
Polynomial P, rear, t1, t2, t;
int c, e;
if (!P1 || !P2) {
return NULL;
}
t1 = P1;
t2 = P2;
P = (Polynomial)malloc(sizeof(struct PolyNode));
P->next = NULL;
rear = P;
while (t2) {
c = t1->coef * t2->coef;
e = t1->expon + t2->expon;
t = (Polynomial)malloc(sizeof(struct PolyNode));
t->coef = c;
t->expon = e;
t->next = NULL;
rear->next = t;
rear = t;
t2 = t2->next;
}
t1 = t1->next;
while (t1) {
t2 = P2;
rear = P;
while (t2) {
e = t1->expon + t2->expon;
c = t1->coef * t2->coef;
while (rear->next && rear->next->expon > e) {
rear = rear->next;
}
if (rear->next && rear->next->expon == e) {
if (rear->next->coef + c) {
rear->next->coef += c;
} else {
t = rear->next;
rear->next = t->next;
free(t);
}
} else {
t = (Polynomial)malloc(sizeof(struct PolyNode));
t->coef = c;
t->expon = e;
t->next = rear->next;
rear->next = t;
rear = rear->next;
}
t2 = t2->next;
}
t1 = t1->next;
}
t2 = P;
P = P->next;
free(t2);
return P;
}
```
7. 实现多项式的求导函数,可以遍历多项式链表并将每一项的指数减1,然后将系数乘以指数,例如:
```
Polynomial Derivative(Polynomial P) {
Polynomial t = P;
while (t) {
t->coef *= t->expon;
t->expon--;
t = t->next;
}
return P;
}
```
相关推荐
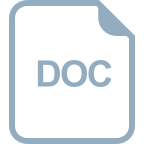













