优化代码newArr.map((item) => { item.dialog.readonly = false; if (data.storageType == 1) { if ( item.key == 'name' || item.key == 'brand' || item.key == 'model' || item.key == 'specification' || item.key == 'unit' ) { item.dialog.disabled = true; } } else { if ( item.key == 'name' || item.key == 'brand' || item.key == 'model' || item.key == 'specification' || item.key == 'unit' ) { item.dialog.disabled = false; } } if (data.type == 2) { if (item.key == 'mainAssetCode') { item.dialog.hide = false; } } else { if (item.key == 'mainAssetCode') { item.dialog.hide = true; } } if (data.storageType == 1) { if (item.key == 'orderCode') { item.dialog.hide = false; } } else { if (item.key == 'orderCode') { item.dialog.hide = true; } } });
时间: 2024-03-20 13:40:39 浏览: 16
可以使用对象解构和简化条件语句来优化上述代码,如下所示:
```
newArr.forEach((item) => {
const { dialog, key } = item;
dialog.readonly = false;
if (data.storageType === 1) {
dialog.disabled = ['name', 'brand', 'model', 'specification', 'unit'].includes(key);
dialog.hide = key === 'orderCode' ? false : dialog.hide;
} else {
dialog.disabled = false;
dialog.hide = key === 'orderCode' ? true : dialog.hide;
}
if (data.type === 2) {
dialog.hide = key === 'mainAssetCode' ? false : dialog.hide;
} else {
dialog.hide = key === 'mainAssetCode' ? true : dialog.hide;
}
});
```
这样做可以使代码更加简洁易读,同时减少重复的代码块。
相关问题
如何得到newArr数组中的companyName并根据数组显示 let groups = [...new Set(company2.map(item => item.parentCode))]; let newArr = groups.map(parentCode =>{ return company2.filter(item => item.parentCode == parentCode); }) this.child = newArr; console.log(this.child)
可以使用数组的 map 方法遍历 newArr 数组,然后在每个子数组中使用 map 方法获取 companyName,最后将其显示出来。可以参考下面的代码示例:
```
let groups = [...new Set(company2.map(item => item.parentCode))];
let newArr = groups.map(parentCode =>{
return company2.filter(item => item.parentCode == parentCode);
});
this.child = newArr;
console.log(this.child);
// 获取 companyName 并显示
this.child.map(group => {
group.map(item => {
console.log(item.companyName);
});
});
```
在上面的代码中,我们首先使用 Set 和 map 方法获取了按 parentCode 分组后的子数组 newArr,然后将其赋值给 this.child,并在控制台中输出了 this.child 的值。
接下来,我们使用 map 方法遍历 this.child,然后使用 map 方法遍历每个子数组 group,最后获取其中的 companyName 并显示出来。
arr.map(item => {
这是一个使用箭头函数定义的回调函数,通常用于Array的map方法。箭头函数是ES6新增的一种语法,用于声明一个匿名函数。箭头函数的语法如下:
```javascript
(parameters) => { statements }
```
其中,parameters是函数的参数列表,可以是0个、1个或多个参数,如果有多个参数,需要用括号括起来;statements是函数的执行体,可以是一条语句或多条语句,如果有多条语句,需要用花括号括起来。
在这个例子中,箭头函数的参数是item,表示数组中的每个元素,箭头函数的执行体是一个代码块,用花括号括起来,这个代码块中可以包含一条或多条语句。在这个例子中,可能是对item进行一些操作,然后返回一个结果,这个结果会被添加到新的数组中,最终函数返回一个新的数组。
需要注意的是,如果箭头函数的执行体只有一条语句,可以省略花括号和return关键字,例如:
```javascript
const arr = [1, 2, 3];
const newArr = arr.map(item => item * 2);
console.log(newArr); // [2, 4, 6]
```
这个例子中,箭头函数的执行体只有一条语句,即item * 2,因此可以省略花括号和return关键字。
相关推荐
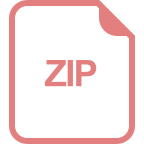
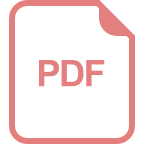
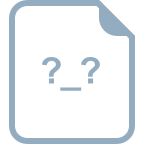













