用C++语言直接写一个代码不要复述文字在主函数中,可以创建一个指向 Book 类的指针数组,然后动态地创建 Book 类的对象和 EBook 类的对象,将这些对象存储到指针数组中。然后,可以通过循环来调用每个对象的 print() 函数,实现多态输出。
时间: 2023-02-16 11:21:13 浏览: 127
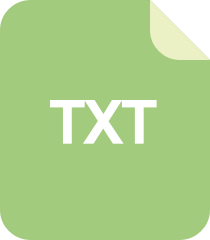
C++多态的使用方法代码,根据实际对象类型来执行适当的操作
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义 Book 类
class Book {
public:
char title[100];
char author[100];
int pages;
// 构造函数
Book(char* title, char* author, int pages) {
strcpy(this->title, title);
strcpy(this->author, author);
this->pages = pages;
}
// 虚函数,用于实现多态
virtual void print() {
printf("Book: %s by %s, %d pages\n", title, author, pages);
}
};
// 定义 EBook 类,继承自 Book 类
class EBook : public Book {
public:
char format[100];
int size;
// 构造函数,调用父类构造函数
EBook(char* title, char* author, int pages, char* format, int size) :
Book(title, author, pages) {
strcpy(this->format, format);
this->size = size;
}
// 覆盖父类的 print() 函数,实现多态
void print() {
printf("EBook: %s by %s, %d pages, %s format, %d MB\n", title, author, pages, format, size);
}
};
int main() {
// 创建指向 Book 类的指针数组
Book* books[3];
// 动态地创建 Book 类的对象和 EBook 类的对象,将这些对象存储到指针数组中
books[0] = new Book("The Great Gatsby", "F. Scott Fitzgerald", 180);
books[1] = new EBook("Moby-Dick", "Herman Melville", 704, "PDF", 2);
books[2] = new EBook("Pride and Prejudice", "Jane Austen", 354, "ePub", 1);
// 通过循环来调用每个对象的 print() 函数,实现多态输出
for (int i = 0; i < 3; i++) {
books[i]->print();
}
return 0;
}
```
阅读全文
相关推荐
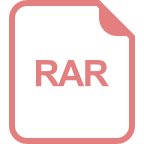




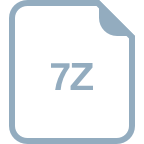
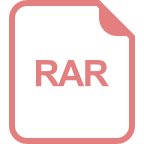
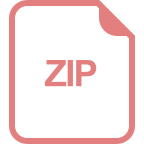
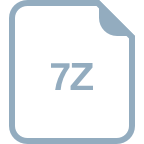
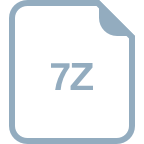
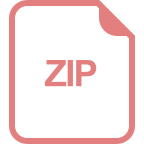
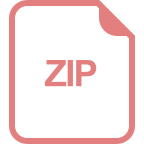
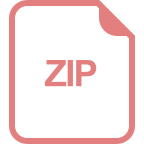
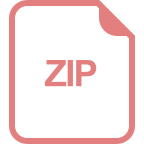