自定义矩阵行数列数左上右上右下坐标,要求用C Shap语言求出矩阵所有坐标
时间: 2023-08-31 08:31:58 浏览: 107
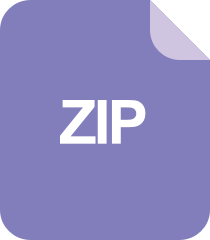
FME经纬度(度分秒)坐标转换为shap矢量图形工具
### 回答1:
以下是一个C#函数,可以根据输入的行数、列数及左上、右上、右下点坐标计算出矩阵中所有点的坐标:
```
public static List<Tuple<int, int>> GetMatrixCoordinates(int rows, int columns, Tuple<int, int> topLeft, Tuple<int, int> topRight, Tuple<int, int> bottomRight)
{
List<Tuple<int, int>> result = new List<Tuple<int, int>>();
// 计算左下点坐标
Tuple<int, int> bottomLeft = new Tuple<int, int>(bottomRight.Item1 - rows + 1, bottomRight.Item2 - columns + 1);
// 遍历矩阵中所有点并加入结果列表
for (int i = topLeft.Item1; i <= bottomLeft.Item1; i++)
{
for (int j = topLeft.Item2; j <= topRight.Item2; j++)
{
result.Add(new Tuple<int, int>(i, j));
}
}
return result;
}
```
使用示例:
```
int rows = 3;
int columns = 4;
Tuple<int, int> topLeft = new Tuple<int, int>(2, 5);
Tuple<int, int> topRight = new Tuple<int, int>(2, 8);
Tuple<int, int> bottomRight = new Tuple<int, int>(4, 8);
List<Tuple<int, int>> coordinates = GetMatrixCoordinates(rows, columns, topLeft, topRight, bottomRight);
foreach (Tuple<int, int> coordinate in coordinates)
{
Console.WriteLine("(" + coordinate.Item1 + ", " + coordinate.Item2 + ")");
}
```
输出结果:
```
(2, 5)
(2, 6)
(2, 7)
(2, 8)
(3, 5)
(3, 6)
(3, 7)
(3, 8)
(4, 5)
(4, 6)
(4, 7)
(4, 8)
```
### 回答2:
在使用C#语言求解矩阵的所有坐标时,我们可以通过自定义矩阵的行数和列数,以及左上、右上和右下的坐标来实现。
首先,我们需要定义一个二维整数数组来表示矩阵,可以使用以下代码进行定义:
int[,] matrix = new int[row, col];
其中,row表示矩阵的行数,col表示矩阵的列数。
接下来,我们可以使用两个嵌套的for循环来遍历矩阵的所有元素,通过打印每一个元素的坐标,即可得到矩阵的所有坐标。具体代码如下:
for (int i = 0; i < row; i++)
{
for (int j = 0; j < col; j++)
{
Console.WriteLine("坐标: ({0}, {1})", i, j);
}
}
其中,i表示矩阵的行号,j表示矩阵的列号。
此外,如果还需要打印左上、右上和右下的坐标,可以根据矩阵的行数和列数进行判断,具体代码如下:
Console.WriteLine("左上坐标: (0, 0)");
Console.WriteLine("右上坐标: (0, {0})", col - 1);
Console.WriteLine("右下坐标: ({0}, {1})", row - 1, col - 1);
其中,col - 1表示矩阵的最后一列的列号,row - 1表示矩阵的最后一行的行号。
通过上述代码,可以求解出矩阵的所有坐标,包括自定义矩阵的行数列数、左上、右上和右下的坐标。
### 回答3:
使用C#语言可以使用循环来求解矩阵的所有坐标。首先,根据给定的行数和列数创建一个二维数组作为矩阵。然后,可以使用两个嵌套的循环来遍历每一个元素,并打印出对应的坐标。
具体代码如下:
```csharp
using System;
class Program
{
static void Main()
{
int rows = 3; // 矩阵的行数
int columns = 4; // 矩阵的列数
int[,] matrix = new int[rows, columns]; // 创建一个行数为rows,列数为columns的矩阵
// 遍历每一个元素,打印出对应的坐标
for (int i = 0; i < rows; i++)
{
for (int j = 0; j < columns; j++)
{
Console.WriteLine("坐标 ({0}, {1})", i, j);
}
}
}
}
```
在上述代码中,我们创建了一个3行4列的矩阵,并使用嵌套循环遍历矩阵的每一个元素,并打印出对应的坐标。你可以根据实际需求调整矩阵的行数、列数和输出形式。
阅读全文
相关推荐
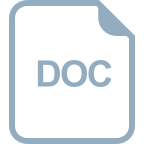
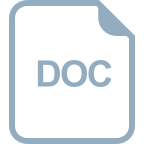



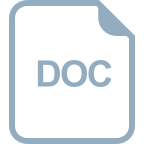
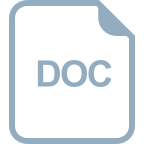
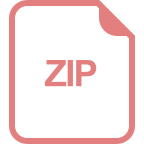
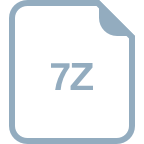
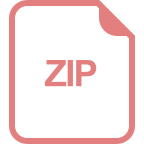
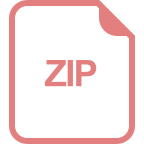
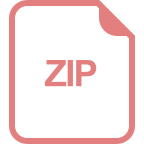
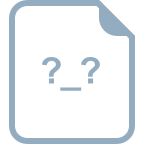
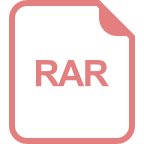
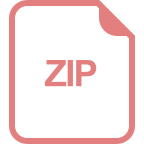
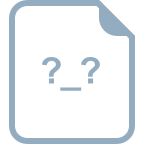
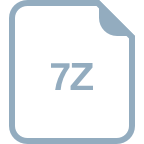
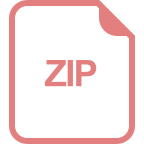