使用STC8051单片机设计一个流水灯,当开关断开时流水灯右移,时间间隔为0.5s;当开关闭合时流水灯左移,时间间隔1s。
时间: 2024-10-28 10:11:09 浏览: 37
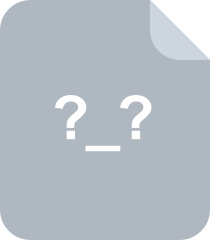
51单片机一个LED流水灯(由8个LED灯组成),单向流水,时间间隔为200ms。

在STC8051单片机中设计一个流水灯系统需要以下几个步骤:
1. **硬件连接**:
- 将数码管作为LED流水灯连接到单片机的P0口,通常每个数码管的7段对应P0的一个位。
- 添加一个按键,如SW1,用于控制灯的移动方向,接至单片机的IO口中,例如P2.0。
2. **软件部分**:
- **定时器设置**:使用单片机的定时器(如Timer1)来实现延时功能。将计数初值设为高优先级模式,比如设置定时器工作于模式1,计数从内部振荡器频率除以128开始计数,以便0.5s或1s的延迟。
```c
#pragma config _XTAL_FREQ = OSC128_PLL ' Oscillator Selection bit (XTAL1 driven by internal oscillator at FOSC/4)
...
unsigned char delay_time;
void timer_init() {
TMOD = 0x01; // Timer1 mode 1 (16-bit timer prescaler x8)
TH1 = 0xFD; // Set initial timer value for 0.5s or 1s depending on the prescaler and clock speed
TL1 = 0xFD;
}
```
- **主循环处理**:
a. 检查按键状态,如果按下则改变计数方向(左移用减法,右移用加法),然后启动定时器。
b. 定时器溢出后,停止定时器并清零,然后控制LED流水灯按照新方向移动一位。
```c
while(1) {
if(SW1 == 0) { // SW1 is pressed
direction = !direction; // Change direction
}
if(!timer_flag) { // If timer hasn't overflowed
timer_flag = 1; // Start timer
} else { // Timer has overflowed
timer_flag = 0; // Stop timer
if(direction) { // Right shift
P0--;
if(P0 == 0xFF) P0 = 0x00; // Wrap around if necessary
} else { // Left shift
P0++;
if(P0 == 0x00) P0 = 0xFF;
}
}
}
```
- **注意**: 确保流水灯的状态更新在中断服务程序(ISR)之外处理,以避免干扰定时器的工作。
阅读全文
相关推荐
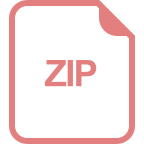
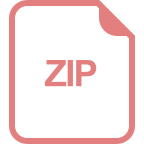
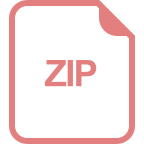
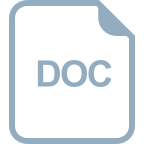
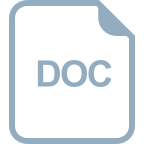
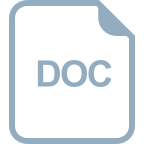
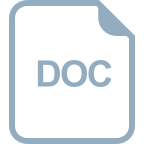
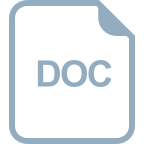
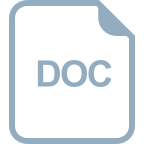
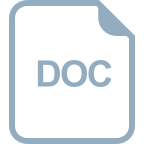
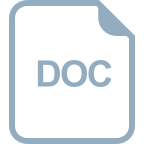




