js字符串转年月日时分秒
时间: 2023-11-11 20:58:44 浏览: 120
可以使用JavaScript内置的Date对象来实现字符串转年月日时分秒的功能。具体实现方法如下:
```javascript
const dateString = '2021-08-31 12:30:45';
const date = new Date(dateString);
const year = date.getFullYear();
const month = date.getMonth() + 1;
const day = date.getDate();
const hour = date.getHours();
const minute = date.getMinutes();
const second = date.getSeconds();
console.log(`${year}-${month}-${day} ${hour}:${minute}:${second}`);
```
输出结果为:`2021-8-31 12:30:45`
相关问题
JavaScript标准时间转年月日时分秒
可以通过 JavaScript 的 Date 对象来实现标准时间到年月日时分秒的转换。具体代码如下:
```javascript
const date = new Date('2022-02-22T22:22:22.222Z');
const year = date.getFullYear(); // 获取年份
const month = date.getMonth() + 1; // 获取月份,需要加 1
const day = date.getDate(); // 获取日期
const hour = date.getHours(); // 获取小时
const minute = date.getMinutes(); // 获取分钟
const second = date.getSeconds(); // 获取秒钟
console.log(`${year}年${month}月${day}日 ${hour}:${minute}:${second}`);
```
运行结果为:
```
2022年2月22日 22:22:22
```
js毫秒数转年月日时分秒
在JavaScript中,将毫秒数转换为年、月、日、时、分、秒,可以通过计算和日期对象的构造函数来实现。以下是一个简单的示例函数,它接受一个毫秒数作为参数:
```javascript
function formatMilliseconds(ms) {
// 将毫秒转换为日期对象
var date = new Date(ms);
// 获取各个部分的值
var years = date.getFullYear();
var months = date.getMonth() + 1; // 注意月份从0开始,加1后才是实际月份
var days = date.getDate();
var hours = date.getHours();
var minutes = date.getMinutes();
var seconds = date.getSeconds();
// 格式化并返回字符串
return {
years: years,
months: padZero(months),
days: padZero(days),
hours: padZero(hours),
minutes: padZero(minutes),
seconds: padZero(seconds)
};
}
// 辅助函数,用于在数字前添加零,保证两位数
function padZero(num) {
return num < 10 ? '0' + num : num;
}
// 使用示例
var milliseconds = 1609459200000; // 某个具体时间的毫秒数(例如2021年1月1日 00:00:00)
var formatted = formatMilliseconds(milliseconds);
console.log(formatted);
```
这个函数会返回一个对象,包含年、月、日、时、分、秒的值,分别用数字表示。注意,月份是从0开始计数的,所以我们加1来获取实际的月份。
阅读全文
相关推荐
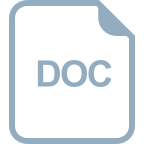
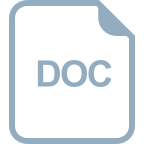
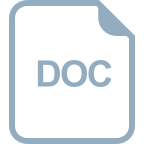












