用方法的重载,实现圆,矩形,三角形的面积
时间: 2024-11-16 11:25:33 浏览: 4
在面向对象编程中,你可以通过方法的重载(Overloading)来实现计算圆、矩形和三角形面积的功能。这里是一个简单的例子,假设我们有一个Shape接口,然后有Circle、Rectangle和Triangle这三个类分别继承自Shape并提供各自的面积计算方法:
```java
// Shape接口
public interface Shape {
double getArea();
}
// Circle类实现了Shape接口
public class Circle implements Shape {
private double radius;
public Circle(double radius) {
this.radius = radius;
}
@Override
public double getArea() {
return Math.PI * radius * radius;
}
}
// Rectangle类也实现Shape接口
public class Rectangle implements Shape {
private double width;
private double height;
public Rectangle(double width, double height) {
this.width = width;
this.height = height;
}
@Override
public double getArea() {
return width * height;
}
}
// Triangle类同样实现Shape接口
public class Triangle implements Shape {
private double base;
private double height;
public Triangle(double base, double height) {
this.base = base;
this.height = height;
}
@Override
public double getArea() {
return 0.5 * base * height; // 使用海伦公式或其他适合三角形的方式
}
}
// 在主函数中,你可以创建任意形状的对象并调用getArea方法来获取面积
public static void main(String[] args) {
Shape circle = new Circle(5);
Shape rectangle = new Rectangle(4, 6);
Shape triangle = new Triangle(3, 7);
System.out.println("Circle area: " + circle.getArea());
System.out.println("Rectangle area: " + rectangle.getArea());
System.out.println("Triangle area: " + triangle.getArea());
}
```
阅读全文
相关推荐
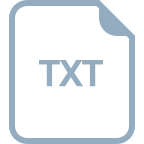
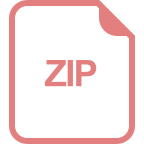
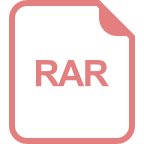















