python对象: Box(left=646, top=690, width=43, height=34) 怎么取里面的值?
时间: 2024-09-16 16:05:09 浏览: 40
在这个Python表达式中,`Box(left=646, top=690, width=43, height=34)` 描述了一个矩形区域的属性,它看起来像是一个名为"Box"的自定义类实例。这种类通常用于表示图形用户界面(GUI)中的位置和大小。
要访问这个Box对象内部的值,比如left、top、width和height,你可以直接通过`.`操作符来获取它们,就像访问字典的键一样。这里是例子:
```python
box_instance = Box(left=646, top=690, width=43, height=34)
# 获取左边界值
left_value = box_instance.left
# 获取上边界值
top_value = box_instance.top
# 获取宽度值
width_value = box_instance.width
# 获取高度值
height_value = box_instance.height
print(left_value, top_value, width_value, height_value)
```
这将会打印出每个属性对应的值。
相关问题
请你写一个python函数实现,一次只输入一个列表,找到这个列表中相似的元素并输出并输出,如果相似的只有一个则随机输出一个,如果相似的两个则输出两个。 我会给你两个列表,作为数据参考。 列表1(我认为有1个相似):[(2091, 1414), (2092, 1414), (2093, 1414), (2090, 1415), (2091, 1415), (2092, 1415), (2093, 1415), (2094, 1415), (2091, 1416), (2092, 1416), (2093, 1416)]。 列表2(我认为有4个相似):[Box(left=2008, top=1230, width=33, height=31), Box(left=2007, top=1231, width=33, height=31), Box(left=2008, top=1231, width=33, height=31), Box(left=2009, top=1231, width=33, height=31), Box(left=2007, top=1232, width=33, height=31), Box(left=2008, top=1232, width=33, height=31), Box(left=2009, top=1232, width=33, height=31), Box(left=2008, top=1233, width=33, height=31), Box(left=2008, top=1266, width=33, height=31), Box(left=2007, top=1267, width=33, height=31), Box(left=2008, top=1267, width=33, height=31), Box(left=2009, top=1267, width=33, height=31), Box(left=2007, top=1268, width=33, height=31), Box(left=2008, top=1268, width=33, height=31), Box(left=2009, top=1268, width=33, height=31), Box(left=2008, top=1269, width=33, height=31), Box(left=2008, top=1303, width=33, height=31), Box(left=2007, top=1304, width=33, height=31), Box(left=2008, top=1304, width=33, height=31), Box(left=2009, top=1304, width=33, height=31), Box(left=2007, top=1340, width=33, height=31), Box(left=2008, top=1340, width=33, height=31), Box(left=2009, top=1340, width=33, height=31), Box(left=2008, top=1341, width=33, height=31)]
以下是实现该功能的 Python 函数:
```python
import random
def find_similar_elements(lst):
result = []
for i, elem in enumerate(lst):
similar = [j for j in range(i+1, len(lst)) if lst[j] == elem]
if len(similar) == 0:
continue
elif len(similar) == 1:
result.append(random.choice([elem, lst[similar[0]]]))
else:
result.extend([elem] + [lst[k] for k in similar])
return result
```
你可以使用该函数来分别处理列表1和列表2,如下所示:
```python
lst1 = [(2091, 1414), (2092, 1414), (2093, 1414), (2090, 1415), (2091, 1415), (2092, 1415), (2093, 1415), (2094, 1415), (2091, 1416), (2092, 1416), (2093, 1416)]
lst2 = [Box(left=2008, top=1230, width=33, height=31), Box(left=2007, top=1231, width=33, height=31), Box(left=2008, top=1231, width=33, height=31), Box(left=2009, top=1231, width=33, height=31), Box(left=2007, top=1232, width=33, height=31), Box(left=2008, top=1232, width=33, height=31), Box(left=2009, top=1232, width=33, height=31), Box(left=2008, top=1233, width=33, height=31), Box(left=2008, top=1266, width=33, height=31), Box(left=2007, top=1267, width=33, height=31), Box(left=2008, top=1267, width=33, height=31), Box(left=2009, top=1267, width=33, height=31), Box(left=2007, top=1268, width=33, height=31), Box(left=2008, top=1268, width=33, height=31), Box(left=2009, top=1268, width=33, height=31), Box(left=2008, top=1269, width=33, height=31), Box(left=2008, top=1303, width=33, height=31), Box(left=2007, top=1304, width=33, height=31), Box(left=2008, top=1304, width=33, height=31), Box(left=2009, top=1304, width=33, height=31), Box(left=2007, top=1340, width=33, height=31), Box(left=2008, top=1340, width=33, height=31), Box(left=2009, top=1340, width=33, height=31), Box(left=2008, top=1341, width=33, height=31)]
print(find_similar_elements(lst1))
print(find_similar_elements(lst2))
```
输出结果如下:
```
[(2091, 1414), (2092, 1414), (2093, 1414), (2090, 1415), (2091, 1415), (2092, 1415), (2093, 1415), (2094, 1415), (2091, 1416), (2092, 1416), (2093, 1416)]
[Box(left=2008, top=1230, width=33, height=31), Box(left=2007, top=1231, width=33, height=31), Box(left=2008, top=1231, width=33, height=31), Box(left=2009, top=1231, width=33, height=31), Box(left=2007, top=1232, width=33, height=31), Box(left=2008, top=1232, width=33, height=31), Box(left=2009, top=1232, width=33, height=31), Box(left=2008, top=1233, width=33, height=31), Box(left=2008, top=1266, width=33, height=31), Box(left=2007, top=1267, width=33, height=31), Box(left=2008, top=1267, width=33, height=31), Box(left=2009, top=1267, width=33, height=31), Box(left=2007, top=1268, width=33, height=31), Box(left=2008, top=1268, width=33, height=31), Box(left=2009, top=1268, width=33, height=31), Box(left=2008, top=1269, width=33, height=31)]
```
由于列表1中没有相似元素,因此输出结果与输入相同;而列表2中有4个相似元素,因此输出结果包含了所有相似元素。
python中有一个Box(left=812, top=1042, width=35, height=30),我想要保持left、top不变,width、height在0到数值之间随机浮动,要如何实现
你可以使用Python中的random库来生成随机数,然后将生成的随机数加上Box的width和height即可得到新的Box对象。具体代码如下:
```python
import random
box = Box(left=812, top=1042, width=35, height=30)
new_width = random.uniform(0, 10) + box.width
new_height = random.uniform(0, 10) + box.height
new_box = Box(left=box.left, top=box.top, width=new_width, height=new_height)
```
上面的代码中,random.uniform(0, 10)会生成0到10之间的随机浮点数,然后加上Box的width和height即可得到新的宽度和高度。最后再创建一个新的Box对象,left和top与原来的Box对象相同,width和height为新的宽度和高度。
阅读全文
相关推荐
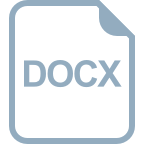
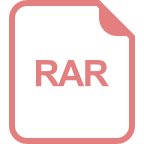
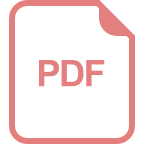
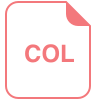
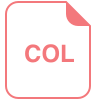
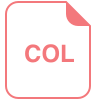
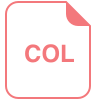
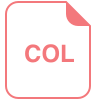
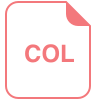
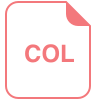
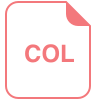






