用java声明圆锥体类,实现 Area 和 Volume 接口,计算表面积和体积,按体积比较大小。如果输入的参数不符合要求(如输入的不是数字等)抛出异常
时间: 2024-10-15 21:09:36 浏览: 27
在Java中,你可以创建一个名为`ConicalShape`的类,它实现`Area`和`Volume`接口,分别用于计算表面积和体积。首先,你需要定义这两个接口:
```java
// 定义Area接口
public interface Area {
double getSurfaceArea();
}
// 定义Volume接口
public interface Volume {
double getVolume();
}
```
然后实现`ConicalShape`类,包括`CircleBase`和`Height`属性,并覆盖接口中的方法:
```java
public class ConicalShape implements Area, Volume {
private double circleBase;
private double height;
public ConicalShape(double base, double height) {
if (base <= 0 || height <= 0) {
throw new IllegalArgumentException("Base and height must be positive numbers.");
}
this.circleBase = base;
this.height = height;
}
// 实现Area接口的方法
@Override
public double getSurfaceArea() {
return Math.PI * circleBase * (circleBase + height);
}
// 实现Volume接口的方法
@Override
public double getVolume() {
return (1.0 / 3.0) * Math.PI * Math.pow(circleBase, 2) * height;
}
// 按照体积比较大小
@Override
public int compareTo(Volume other) {
if (this instanceof Cone && other instanceof Cone) {
return Double.compare(this.getVolume(), other.getVolume());
} else {
throw new ClassCastException("Cannot compare objects of different types for volume comparison");
}
}
// 输入验证方法,只针对构造函数
// ...
}
```
在这个例子中,当你尝试创建`ConicalShape`实例时,如果提供的基础半径或高度不是正数,会抛出`IllegalArgumentException`。
阅读全文
相关推荐
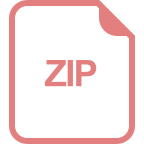
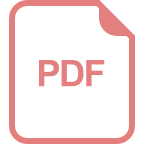
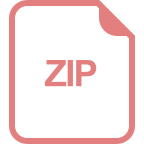



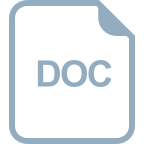











