python如何在每次循环中实时更新lineEdit的文本
时间: 2024-10-28 08:11:24 浏览: 9
在Python中,特别是在PyQt或Tkinter这样的GUI库中,为了实现在循环中实时更新QLineEdit(在PyQt中)或Entry(在Tkinter中)的内容,你可以使用循环配合`time.sleep()`函数模拟定时,并在循环体内部更改元素的文本。以下是一个基本的例子,使用了PyQt5:
```python
import sys
from PyQt5.QtWidgets import QApplication, QWidget, QLabel, QLineEdit
from PyQt5.QtCore import QTimer, QEventLoop
class UpdateApp(QWidget):
def __init__(self):
super().__init__()
self.line_edit = QLineEdit()
self.label = QLabel("实时更新:")
self.update_timer = QTimer(self)
self.update_counter = 0
layout = QVBoxLayout()
layout.addWidget(self.label)
layout.addWidget(self.line_edit)
self.setLayout(layout)
self.update_timer.timeout.connect(self.update_text)
self.update_timer.start(1000) # 每秒更新一次
def update_text(self):
"""在每次定时器触发时更新行编辑器和标签的内容"""
self.update_counter += 1
self.line_edit.setText(f"这是实时更新的第 {self.update_counter} 次")
self.label.setText(f"实时更新:{self.line_edit.text()}")
if __name__ == "__main__":
app = QApplication(sys.argv)
window = UpdateApp()
window.show()
# 使用事件循环等待用户交互和定时器完成
event_loop = QEventLoop()
app.aboutToQuit.connect(event_loop.quit)
try:
event_loop.exec_()
except KeyboardInterrupt:
print("用户中断")
```
在这个例子中,我们创建了一个名为`update_text`的方法,在其中增加计数并在每秒更新`line_edit`的文本。当应用程序接收到`aboutToQuit`信号(即将退出前)时,我们会结束事件循环。
如果你正在使用Tkinter,过程类似,只是不需要使用`QTimer`,而是通过`after`函数:
```python
import tkinter as tk
def update_entry():
entry.delete(0, "end") # 清空
entry.insert(tk.END, f"这是实时更新的第 {counter} 次")
counter += 1
root.after(1000, update_entry) # 每隔1秒执行update_entry
root = tk.Tk()
entry = tk.Entry(root)
counter = 0
update_entry() # 开始第一个更新
root.mainloop()
```
在这个Tkinter版本中,`after`用于设置延时回调函数。
阅读全文
相关推荐
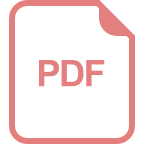
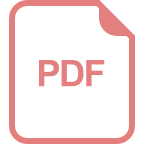










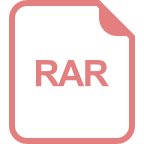
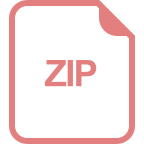
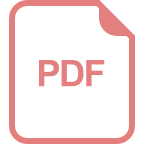
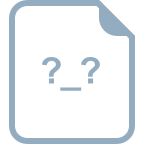
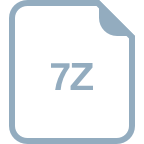
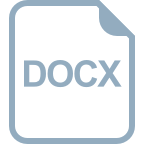