写一段C++代码,要求如下【问题描述】编写先序遍历的非递归算法,显示二叉树的先序序列 【输入形式】AB##C## 【输出形式】ABC 【样例输入】AB##C## 【样例输出】ABC
时间: 2023-10-08 11:10:26 浏览: 28
以下是代码实现:
```c++
#include <iostream>
#include <stack>
using namespace std;
struct TreeNode {
char val;
TreeNode* left;
TreeNode* right;
TreeNode(char x) : val(x), left(nullptr), right(nullptr) {}
};
void preOrder(TreeNode* root) {
if (root == nullptr) {
return;
}
stack<TreeNode*> s;
s.push(root);
while (!s.empty()) {
TreeNode* curr = s.top();
s.pop();
cout << curr->val;
if (curr->right != nullptr) {
s.push(curr->right);
}
if (curr->left != nullptr) {
s.push(curr->left);
}
}
}
int main() {
string input;
cin >> input;
int n = input.size();
stack<TreeNode*> s;
TreeNode* root = new TreeNode(input[0]);
s.push(root);
for (int i = 1; i < n; i++) {
TreeNode* curr = s.top();
if (input[i] == '#') {
s.pop();
} else {
TreeNode* node = new TreeNode(input[i]);
if (curr->left == nullptr) {
curr->left = node;
} else {
curr->right = node;
}
s.push(node);
}
}
preOrder(root);
return 0;
}
```
首先输入一个字符串,代表二叉树的先序遍历序列。通过栈的方式构建二叉树,然后进行非递归的先序遍历,输出先序序列。
相关推荐
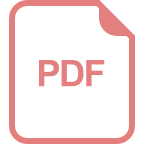














