c++ FaceNet 人脸识别 的程序
时间: 2024-05-01 19:22:02 浏览: 11
人脸识别技术在计算机视觉领域中是非常重要的一个分支,而FaceNet是一种非常流行的人脸识别算法。在C++中实现FaceNet人脸识别需要以下步骤:
1. 下载FaceNet模型
FaceNet模型是由Google开发的深度学习模型,可以通过TensorFlow Hub获取。可以使用以下代码下载模型:
```
#include <tensorflow/core/public/version.h>
#include <tensorflow/core/public/session.h>
#include <tensorflow/core/platform/env.h>
#include <tensorflow/core/graph/default_device.h>
#include <tensorflow/core/framework/tensor.h>
#include <tensorflow/core/framework/tensor_shape.h>
#include <tensorflow/core/framework/types.h>
#include <tensorflow/core/framework/graph.pb.h>
#include <tensorflow/cc/ops/standard_ops.h>
#include <tensorflow/cc/framework/ops.h>
#include <tensorflow/cc/saved_model/loader.h>
#include <iostream>
void downloadFacenetModel(std::string modelPath)
{
tensorflow::Session* session;
tensorflow::Status status = tensorflow::NewSession(tensorflow::SessionOptions(), &session);
if (!status.ok()) {
std::cout << status.ToString() << "\n";
return;
}
tensorflow::MetaGraphDef graph_def;
status = tensorflow::LoadSavedModel(tensorflow::SessionOptions(), tensorflow::RunOptions(), modelPath, {"serve"}, &graph_def);
if (!status.ok()) {
std::cout << status.ToString() << "\n";
return;
}
tensorflow::GraphDef graph;
graph.ToProto(graph_def.graph_def());
status = session->Create(graph_def.graph_def());
if (!status.ok()) {
std::cout << status.ToString() << "\n";
return;
}
std::cout << "Facenet model downloaded successfully!\n";
}
```
2. 加载图片
可以使用OpenCV库加载图片,以下是一个简单的加载图片的示例代码:
```
#include <opencv2/core/core.hpp>
#include <opencv2/highgui/highgui.hpp>
#include <opencv2/imgproc/imgproc.hpp>
cv::Mat loadImage(std::string imagePath)
{
cv::Mat image = cv::imread(imagePath, cv::IMREAD_COLOR);
if (!image.data) {
std::cout << "Could not open or find the image!\n";
return cv::Mat();
}
cv::cvtColor(image, image, cv::COLOR_BGR2RGB);
return image;
}
```
3. 预处理图片
FaceNet需要输入固定大小的图片,而且需要进行归一化。以下是一个简单的预处理图片的示例代码:
```
#include <opencv2/core/core.hpp>
#include <opencv2/imgproc/imgproc.hpp>
cv::Mat preprocessImage(cv::Mat image, int image_size)
{
cv::Mat resized_image;
cv::resize(image, resized_image, cv::Size(image_size, image_size));
resized_image.convertTo(resized_image, CV_32FC3);
resized_image = (resized_image - 127.5) / 128.0;
return resized_image;
}
```
4. 运行FaceNet模型
以下是一个简单的运行FaceNet模型的示例代码:
```
#include <tensorflow/cc/ops/standard_ops.h>
#include <tensorflow/cc/framework/ops.h>
std::vector<float> runFacenetModel(cv::Mat image, tensorflow::Session* session, int image_size)
{
std::vector<tensorflow::Tensor> outputs;
tensorflow::Tensor input_tensor(tensorflow::DT_FLOAT, tensorflow::TensorShape({1, image_size, image_size, 3}));
float* tensor_data = input_tensor.flat<float>().data();
memcpy(tensor_data, image.data, image.total() * image.elemSize());
tensorflow::ops::Placeholder input_placeholder(tensorflow::ops::Placeholder::Shape({1, image_size, image_size, 3}));
tensorflow::ops::Identity identity("input", input_placeholder);
std::vector<std::pair<std::string, tensorflow::Tensor>> inputs = {{"input:0", input_tensor}};
tensorflow::Status status = session->Run(inputs, {"embeddings"}, {}, &outputs);
if (!status.ok()) {
std::cout << status.ToString() << "\n";
return std::vector<float>();
}
std::vector<float> embeddings;
float* embeddings_data = outputs[0].flat<float>().data();
for (int i = 0; i < outputs[0].shape().dim_size(1); i++) {
embeddings.push_back(embeddings_data[i]);
}
return embeddings;
}
```
完整的人脸识别程序需要将以上步骤结合起来,可以参考以下的示例代码:
```
#include <opencv2/core/core.hpp>
#include <opencv2/highgui/highgui.hpp>
#include <opencv2/imgproc/imgproc.hpp>
#include <tensorflow/core/public/version.h>
#include <tensorflow/core/public/session.h>
#include <tensorflow/core/platform/env.h>
#include <tensorflow/core/graph/default_device.h>
#include <tensorflow/core/framework/tensor.h>
#include <tensorflow/core/framework/tensor_shape.h>
#include <tensorflow/core/framework/types.h>
#include <tensorflow/core/framework/graph.pb.h>
#include <tensorflow/cc/ops/standard_ops.h>
#include <tensorflow/cc/framework/ops.h>
#include <tensorflow/cc/saved_model/loader.h>
#include <iostream>
void downloadFacenetModel(std::string modelPath)
{
tensorflow::Session* session;
tensorflow::Status status = tensorflow::NewSession(tensorflow::SessionOptions(), &session);
if (!status.ok()) {
std::cout << status.ToString() << "\n";
return;
}
tensorflow::MetaGraphDef graph_def;
status = tensorflow::LoadSavedModel(tensorflow::SessionOptions(), tensorflow::RunOptions(), modelPath, {"serve"}, &graph_def);
if (!status.ok()) {
std::cout << status.ToString() << "\n";
return;
}
tensorflow::GraphDef graph;
graph.ToProto(graph_def.graph_def());
status = session->Create(graph_def.graph_def());
if (!status.ok()) {
std::cout << status.ToString() << "\n";
return;
}
std::cout << "Facenet model downloaded successfully!\n";
}
cv::Mat loadImage(std::string imagePath)
{
cv::Mat image = cv::imread(imagePath, cv::IMREAD_COLOR);
if (!image.data) {
std::cout << "Could not open or find the image!\n";
return cv::Mat();
}
cv::cvtColor(image, image, cv::COLOR_BGR2RGB);
return image;
}
cv::Mat preprocessImage(cv::Mat image, int image_size)
{
cv::Mat resized_image;
cv::resize(image, resized_image, cv::Size(image_size, image_size));
resized_image.convertTo(resized_image, CV_32FC3);
resized_image = (resized_image - 127.5) / 128.0;
return resized_image;
}
std::vector<float> runFacenetModel(cv::Mat image, tensorflow::Session* session, int image_size)
{
std::vector<tensorflow::Tensor> outputs;
tensorflow::Tensor input_tensor(tensorflow::DT_FLOAT, tensorflow::TensorShape({1, image_size, image_size, 3}));
float* tensor_data = input_tensor.flat<float>().data();
memcpy(tensor_data, image.data, image.total() * image.elemSize());
tensorflow::ops::Placeholder input_placeholder(tensorflow::ops::Placeholder::Shape({1, image_size, image_size, 3}));
tensorflow::ops::Identity identity("input", input_placeholder);
std::vector<std::pair<std::string, tensorflow::Tensor>> inputs = {{"input:0", input_tensor}};
tensorflow::Status status = session->Run(inputs, {"embeddings"}, {}, &outputs);
if (!status.ok()) {
std::cout << status.ToString() << "\n";
return std::vector<float>();
}
std::vector<float> embeddings;
float* embeddings_data = outputs[0].flat<float>().data();
for (int i = 0; i < outputs[0].shape().dim_size(1); i++) {
embeddings.push_back(embeddings_data[i]);
}
return embeddings;
}
float calculateDistance(std::vector<float> embedding1, std::vector<float> embedding2)
{
float distance = 0;
for (int i = 0; i < embedding1.size(); i++) {
distance += pow((embedding1[i] - embedding2[i]), 2);
}
distance = sqrt(distance);
return distance;
}
int main()
{
std::string modelPath = "facenet_model";
downloadFacenetModel(modelPath);
tensorflow::Session* session;
tensorflow::Status status = tensorflow::NewSession(tensorflow::SessionOptions(), &session);
if (!status.ok()) {
std::cout << status.ToString() << "\n";
return 0;
}
tensorflow::MetaGraphDef graph_def;
status = tensorflow::LoadSavedModel(tensorflow::SessionOptions(), tensorflow::RunOptions(), modelPath, {"serve"}, &graph_def);
if (!status.ok()) {
std::cout << status.ToString() << "\n";
return 0;
}
tensorflow::GraphDef graph;
graph.ToProto(graph_def.graph_def());
status = session->Create(graph_def.graph_def());
if (!status.ok()) {
std::cout << status.ToString() << "\n";
return 0;
}
std::string image1Path = "image1.jpg";
std::string image2Path = "image2.jpg";
cv::Mat image1 = loadImage(image1Path);
cv::Mat image2 = loadImage(image2Path);
int image_size = 160;
cv::Mat resized_image1 = preprocessImage(image1, image_size);
cv::Mat resized_image2 = preprocessImage(image2, image_size);
std::vector<float> embedding1 = runFacenetModel(resized_image1, session, image_size);
std::vector<float> embedding2 = runFacenetModel(resized_image2, session, image_size);
float distance = calculateDistance(embedding1, embedding2);
std::cout << "Distance: " << distance << "\n";
return 0;
}
```
在运行这个程序之前,需要先下载FaceNet模型,并将模型保存到"facenet_model"目录下。完整的程序需要两张人脸图片作为输入,然后输出这两张人脸图片之间的距离。
相关推荐
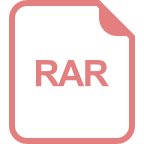
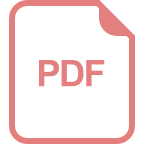














