{% extends 'base.html' %} {% block title %}修改已退备注{% endblock %} {% block main %} <html> <head> <style> input[type="text"] { resize: both; } </style> </head> <body id="body"> <div id="left_side"> <ul> <li>修改已退备注</li> </ul> </div> <div id="right_side"> <form action="/do_change_yitui" method="post"> <!-- 定义一个多行的文本输入--> 已选择的运单号: <br> <input type="text" name="yundanhao" value="{{ids}}" > <br> <br> <input type="text" name="beizhu" placeholder="点此输入备注"> <br> <br> <input type="submit" value="提交"> </form> </div> </body> </html> {% endblock %}为什么css不生效
时间: 2023-10-25 11:08:54 浏览: 99
根据您提供的代码,CSS样式文件中的样式可能不会生效,因为您没有将它们包含在`<style>`标记中。这是因为您使用了Django模板语言,而`<style>`标记在模板文件中被视为普通文本,因此CSS样式不会被正确解析。
要使CSS样式生效,请将它们放置在base.html文件中的`<head>`标记中,例如:
```
<!DOCTYPE html>
<html>
<head>
<title>{% block title %}{% endblock %}</title>
<style>
input[type="text"] {
resize: both;
}
</style>
</head>
<body>
{% block main %}{% endblock %}
</body>
</html>
```
然后在您的模板文件中,使用`{% extends %}`指令扩展base.html文件,并在`{% block main %}`标记中包含您的页面内容。这样,您的CSS样式将被正确加载并应用于页面。
相关问题
如何在django中用django-redis实现网页输入数据redis接受储存数据,网页的代码如下{% extends './base.html' %} {% block base %} <div class="main"> <div class="title-box" style="color:#444;"> <h3>新增学生</h3> </div> <div class="form-box"> {% if messages %} {% for message in messages %} <div class="alert alert-danger" role="alert" style="width: 70%">{{ message }}</div> {% endfor %} {% endif %} <div class="row"> <div class="col-5"> <form action="{% url 'card:student_create' %}" method="POST"> {% csrf_token %} <div class="form-group"> <label>学生学号</label> <input type="text" name="student_code" class="form-control"> <small class="form-text text-muted"></small> </div> <div class="form-group"> <label>学生姓名</label> <input type="text" name="name" class="form-control"> <small class="form-text text-muted"></small> </div> <div class="form-group"> <label>学生电话</label> <input type="text" name="phone" class="form-control"> <small class="form-text text-muted"></small> </div> <div class="form-group"> <label>学生地址</label> <input type="text" name="address" class="form-control"> <small class="form-text text-muted"></small> </div> <div class="form-group"> <label>学生院系</label> <input type="text" name="faculty" class="form-control"> <small class="form-text text-muted"></small> </div> <div class="form-group"> <label>学生专业</label> <input type="text" name="major" class="form-control"> <small class="form-text text-muted"></small> </div> <div class="submit-box"> <button type="submit" class="btn btn-primary" style="background: #18a5db; border-color: #18a5db;">提交 <tton> </div> </form> </div> </div> </div> </div> {% endblock base %} 该如何写
首先,需要安装django-redis和redis模块。可以使用以下命令:
```
pip install django-redis redis
```
然后,在Django的settings.py文件中配置redis数据库:
```
CACHES = {
'default': {
'BACKEND': 'django_redis.cache.RedisCache',
'LOCATION': 'redis://127.0.0.1:6379/1',
'OPTIONS': {
'CLIENT_CLASS': 'django_redis.client.DefaultClient',
}
}
}
```
接着,在views.py文件中,可以使用以下代码将数据存储到redis数据库中:
```
from django.shortcuts import render
from django_redis import get_redis_connection
def student_create(request):
if request.method == 'POST':
student_code = request.POST.get('student_code')
name = request.POST.get('name')
phone = request.POST.get('phone')
address = request.POST.get('address')
faculty = request.POST.get('faculty')
major = request.POST.get('major')
# 将数据存储到redis数据库中
redis_conn = get_redis_connection()
redis_conn.set(student_code, f'{name},{phone},{address},{faculty},{major}')
return render(request, 'student_create.html')
```
在上面的代码中,我们通过get_redis_connection()方法获取redis连接对象,然后使用set()方法将数据存储到redis数据库中。其中,学生学号作为key,学生姓名、电话、地址、院系、专业等信息组成的字符串作为value。
最后,在urls.py文件中配置对应的路由:
```
from django.urls import path
from .views import student_create
app_name = 'card'
urlpatterns = [
path('student/create/', student_create, name='student_create'),
]
```
这样,在网页中输入数据并提交后,数据就会被存储到redis数据库中了。
flask中用html嵌套多个html的高阶方法
在Flask中,可以使用模板继承的方式来嵌套多个HTML文件。模板继承是一种将一个基础模板与其他模板结合使用的技术,这样可以在基础模板中定义通用的结构和样式,然后在其他模板中使用该结构和样式,并且可以在其他模板中添加自己的内容。
下面是一个简单的例子:
1. 创建一个基础模板base.html,其中包含通用的结构和样式:
```
<!DOCTYPE html>
<html>
<head>
<title>{% block title %}{% endblock %}</title>
</head>
<body>
<header>
{% block header %}{% endblock %}
</header>
<main>
{% block content %}{% endblock %}
</main>
<footer>
{% block footer %}{% endblock %}
</footer>
</body>
</html>
```
2. 创建一个其他模板home.html,继承于基础模板,并添加自己的内容:
```
{% extends "base.html" %}
{% block title %}Home{% endblock %}
{% block header %}
<h1>Welcome to my website</h1>
{% endblock %}
{% block content %}
<p>This is the home page.</p>
{% endblock %}
```
3. 创建另一个其他模板about.html,同样继承于基础模板,并添加自己的内容:
```
{% extends "base.html" %}
{% block title %}About{% endblock %}
{% block header %}
<h1>About us</h1>
{% endblock %}
{% block content %}
<p>We are a company that specializes in web development.</p>
{% endblock %}
```
在这个例子中,我们使用了Flask的模板引擎Jinja2来实现模板继承。在基础模板中,我们使用了Jinja2的block语句来定义可替换的块,而在其他模板中,我们使用了extends语句来继承基础模板,并使用block语句来定义自己的内容。
最后,我们可以在Flask应用程序中渲染这些模板,如下所示:
```
from flask import Flask, render_template
app = Flask(__name__)
@app.route('/')
def home():
return render_template('home.html')
@app.route('/about')
def about():
return render_template('about.html')
if __name__ == '__main__':
app.run()
```
这样,当我们访问网站的根目录时,会显示home.html模板中的内容,而当我们访问/about时,则会显示about.html模板中的内容。同时,两个页面都包含了基础模板中的通用结构和样式。
阅读全文
相关推荐
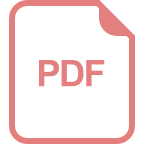
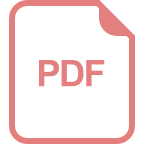
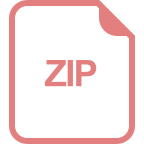
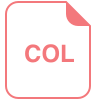
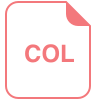
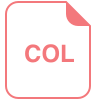
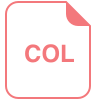
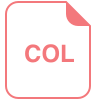
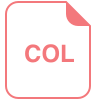
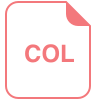
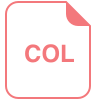
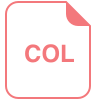
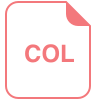
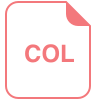
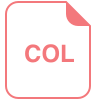
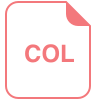
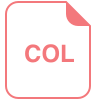