编写一个c语言程序,给定一个字符串数组words,手动输入字符串s,输出words中是s子序列的字符串个数
时间: 2024-10-20 07:12:37 浏览: 38
编写一个C语言程序来统计给定字符串数组words中包含指定字符串s的子序列的数量,可以采用动态规划的方法。首先,定义一个辅助函数`isSubsequence()`判断s是否是words中的某个字符串的子序列,然后在主函数中遍历数组并计数。以下是简单实现:
```c
#include <stdio.h>
#include <string.h>
// 辅助函数,判断s是否是str的子序列
int isSubsequence(char* s, char* str) {
int i = 0, j = 0;
while (i < strlen(s) && j < strlen(str)) {
if (s[i] == str[j]) {
i++;
}
j++;
}
return i == strlen(s);
}
// 主函数,计算子序列个数
int countSubsequences(char** words, int n, char* s) {
int count = 0;
for (int i = 0; i < n; i++) {
if (isSubsequence(s, words[i])) {
count++;
}
}
return count;
}
int main() {
int num_words;
printf("Enter the number of strings in the array: ");
scanf("%d", &num_words);
char** word_array = (char**)malloc(num_words * sizeof(char*));
if (word_array == NULL) {
printf("Memory allocation failed.\n");
return 1;
}
printf("Enter the strings in the array (separated by space): ");
for (int i = 0; i < num_words; i++) {
word_array[i] = malloc(strlen(NULL) + 1); // Add space for null terminator
fgets(word_array[i], sizeof(word_array[i]), stdin);
word_array[i][strlen(word_array[i])-1] = '\0'; // Remove newline character at the end
}
char input_s[100];
printf("Enter the string to find subsequences: ");
fgets(input_s, sizeof(input_s), stdin);
input_s[strlen(input_s)-1] = '\0'; // Remove newline character
int result = countSubsequences(word_array, num_words, input_s);
printf("Number of strings in the array that have '%s' as a subsequence: %d\n", input_s, result);
// Free memory
for (int i = 0; i < num_words; i++) {
free(word_array[i]);
}
free(word_array);
return 0;
}
```
阅读全文
相关推荐
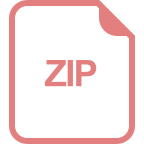
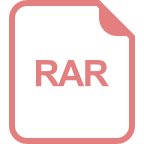
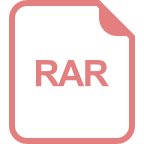
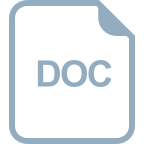














