easypoi批量导入excel文件的数据 返回校验失败的数据写入excel的文件
时间: 2024-05-16 21:15:34 浏览: 139
要使用 Easypoi 实现批量导入 Excel 数据并返回校验失败的数据,并将其写入 Excel 文件,可以按照以下步骤进行操作:
1. 定义一个 Excel 实体类,用于存储导入的 Excel 数据,同时添加校验注解,如下所示:
```java
public class UserExcelEntity implements Serializable {
@Excel(name = "姓名", orderNum = "0")
@NotBlank(message = "姓名不能为空")
private String name;
@Excel(name = "年龄", orderNum = "1")
@Range(min = 1, max = 200, message = "年龄范围必须在1-200之间")
private Integer age;
// ... 其他属性和校验注解
}
```
2. 定义一个导入工具类,使用 Easypoi 实现批量导入 Excel 数据,并返回校验失败的数据,如下所示:
```java
public class ExcelImportUtil {
public static <T> List<T> importExcel(MultipartFile file, Class<T> clazz, List<String> errorMsg) throws Exception {
List<T> successList = new ArrayList<>();
List<T> errorList = new ArrayList<>();
ImportParams params = new ImportParams();
params.setTitleRows(1);
params.setHeadRows(1);
ExcelImportResult<T> result = ExcelImportUtil.importExcelMore(file.getInputStream(), clazz, params);
List<T> list = result.getList();
for (T obj : list) {
Set<ConstraintViolation<T>> validateSet = Validation.buildDefaultValidatorFactory().getValidator().validate(obj);
if (validateSet.isEmpty()) {
successList.add(obj);
} else {
errorMsg.add(buildErrorMsg(validateSet));
errorList.add(obj);
}
}
if (!errorList.isEmpty()) {
String fileName = "error_" + System.currentTimeMillis() + ".xlsx";
String filePath = "D:/excel/" + fileName;
ExportParams exportParams = new ExportParams();
Workbook workbook = ExcelExportUtil.exportExcel(exportParams, clazz, errorList);
FileOutputStream fos = new FileOutputStream(filePath);
workbook.write(fos);
fos.close();
}
return successList;
}
private static <T> String buildErrorMsg(Set<ConstraintViolation<T>> validateSet) {
StringBuilder sb = new StringBuilder();
for (ConstraintViolation<T> validate : validateSet) {
sb.append(validate.getPropertyPath().toString()).append(":").append(validate.getMessage()).append(";");
}
return sb.toString();
}
}
```
3. 在Controller中调用导入工具类,将校验失败的数据写入 Excel 文件,并返回校验成功的数据,如下所示:
```java
@PostMapping("/import")
public Result importExcel(@RequestParam("file") MultipartFile file) throws Exception {
List<String> errorMsg = new ArrayList<>();
List<UserExcelEntity> list = ExcelImportUtil.importExcel(file, UserExcelEntity.class, errorMsg);
if (!errorMsg.isEmpty()) {
return Result.error("部分数据导入失败,请下载错误文件查看详情!");
}
// 保存校验成功的数据
userService.saveBatch(list);
return Result.success("数据导入成功!");
}
```
其中,将校验失败的数据写入 Excel 文件的代码如下:
```java
if (!errorList.isEmpty()) {
String fileName = "error_" + System.currentTimeMillis() + ".xlsx";
String filePath = "D:/excel/" + fileName;
ExportParams exportParams = new ExportParams();
Workbook workbook = ExcelExportUtil.exportExcel(exportParams, clazz, errorList);
FileOutputStream fos = new FileOutputStream(filePath);
workbook.write(fos);
fos.close();
}
```
这里使用了 Easypoi 的 `ExcelExportUtil.exportExcel()` 方法,将校验失败的数据导出为 Excel 文件。
阅读全文
相关推荐
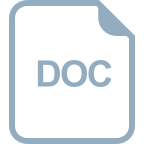
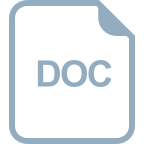
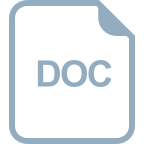

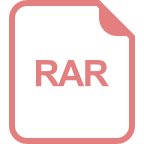
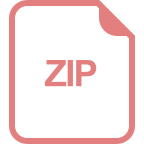
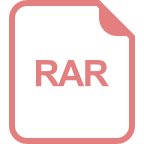
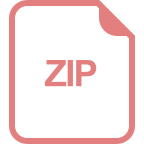
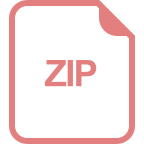
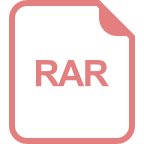
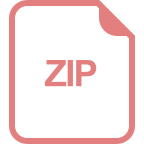
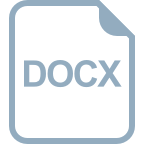
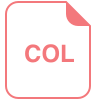

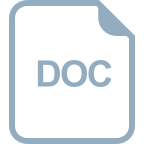
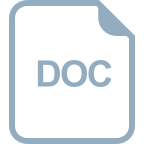
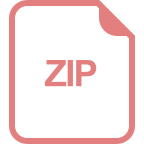
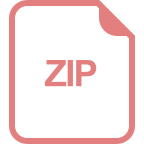
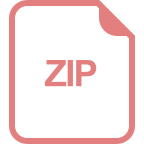
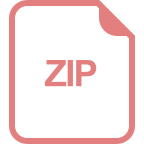