classification = np.where(r == np.amax(r))[1][0],解释
时间: 2024-06-03 21:08:47 浏览: 6
这行代码是用于找到数组r中最大值的索引,这个索引被用来作为分类的标签。具体解释如下:
- np.where(r == np.amax(r)):找到数组r中所有最大值的索引。np.amax(r)返回r中的最大值,r == np.amax(r)返回一个布尔数组,其中True表示对应的元素等于最大值,False表示不等于最大值。np.where()函数返回True元素的索引。
- [1][0]:从返回的元组中取出第二个元素,并从中取出第一个元素。这个元素是最大值的索引,也就是我们要的分类标签。注意,这个代码假设r是一个二维数组,其中每一行都代表一个分类的得分。第一维对应的是样本,第二维对应的是分类。
相关问题
label=np.where(label>1,1,0)
This line of code is using the NumPy library's where function to create a binary label array. It checks if each element in the input array 'label' is greater than 1; if it is, that element is replaced with 1, otherwise it is replaced with 0. The resulting array is returned and assigned to the variable 'label'.
Overall, this code is meant to convert a multi-class classification problem into a binary classification problem, where the positive class is represented by elements greater than 1 in the original label array.
import numpy as np import cv2 as cv import matplotlib.pyplot as plt a = np.random.randint(90,96,size=(20,2)).astype(np.float32) b = np.random.randint(95,101,size=(20,2)).astype(np.float32) data = np.vstack((a,b)) data = np.array(data,dtype=np.float32) a_label = np.zeros((20,1)) b_label = np.ones((20,1)) label = np.vstack((a_label,b_label)) label = np.array(label,dtype=np.float32) svm = cv.ml.SVM_create() svm.setType(cv.ml.SVM_C_SVC) svm.setKernel(cv.ml.SVM_LINEAR) svm.setC(0.01) result = svm.train(data,cv.ml.ROW_SAMPLE,label) test = np.array([[93.92, 96.97]], dtype=np.float32) p1, p2 = svm.predict(test) plt.scatter(a[:,0],a[:,1],s=80,c="g",marker="o") plt.scatter(b[:,0],b[:,1],s=80,c="b",marker="s") plt.scatter(test[:,0],test[:,1],s=80,c="r",marker="*") plt.show() print(p1, p2)为什么代码会报下列错误n the case of classification problem the responses must be categorical; either specify varType when creating TrainData, or pass integer responses in function 'cv::ml::SVMImpl::train'
这个错误说明在训练SVM模型时,响应变量(即标签)不是分类变量,而是连续变量或其他类型的变量。因此,需要将响应变量转换为分类变量才能训练SVM模型。
为了解决这个问题,您需要将标签变量转换为分类变量。您可以使用OpenCV的ml.TrainData()函数来创建训练数据对象,并将响应变量的类型指定为CV_VAR_CATEGORICAL。具体来说,您可以在创建训练数据对象时指定varType参数,如下所示:
```python
train_data = cv.ml.TrainData_create(data, cv.ml.ROW_SAMPLE, label)
train_data.setVarType(np.array([cv.ml.VAR_NUMERICAL, cv.ml.VAR_NUMERICAL], dtype=np.uint8))
```
在这个例子中,我们将响应变量的类型设置为CV_VAR_CATEGORICAL,将特征变量的类型设置为CV_VAR_NUMERICAL,因为特征变量是连续变量。
下面是修改后的代码:
```python
import numpy as np
import cv2 as cv
import matplotlib.pyplot as plt
a = np.random.randint(90,96,size=(20,2)).astype(np.float32)
b = np.random.randint(95,101,size=(20,2)).astype(np.float32)
data = np.vstack((a,b))
data = np.array(data,dtype=np.float32)
a_label = np.zeros((20,1))
b_label = np.ones((20,1))
label = np.vstack((a_label,b_label))
label = np.array(label,dtype=np.float32)
train_data = cv.ml.TrainData_create(data, cv.ml.ROW_SAMPLE, label)
train_data.setVarType(np.array([cv.ml.VAR_NUMERICAL, cv.ml.VAR_NUMERICAL], dtype=np.uint8))
svm = cv.ml.SVM_create()
svm.setType(cv.ml.SVM_C_SVC)
svm.setKernel(cv.ml.SVM_LINEAR)
svm.setC(0.01)
result = svm.train(train_data)
test = np.array([[93.92, 96.97]], dtype=np.float32)
p1, p2 = svm.predict(test)
plt.scatter(a[:,0],a[:,1],s=80,c="g",marker="o")
plt.scatter(b[:,0],b[:,1],s=80,c="b",marker="s")
plt.scatter(test[:,0],test[:,1],s=80,c="r",marker="*")
plt.show()
print(p1, p2)
```
希望这个修改可以帮助您解决问题!
相关推荐
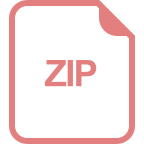
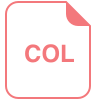
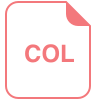
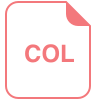
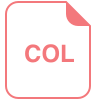
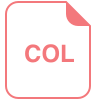








