数据结构带尾指针的单循环链表如何定义,如何创建用c语言的方法
时间: 2024-10-08 08:19:35 浏览: 93
数据结构中带尾指针的单循环链表是一种特殊类型的链表,其中最后一个节点除了指向下一个节点外,还额外有一个指针指向头节点,形成一个闭环。这使得遍历整个列表变得更加简单,同时也便于进行插入和删除操作。
在 C 语言中,可以这样定义一个带尾指针的单循环链表节点:
```c
typedef struct Node {
int data; // 节点存储的数据
struct Node* next; // 指向下一个节点的指针
struct Node* tail; // 指向头节点的尾部指针
} ListNode;
```
创建这个链表并初始化的方法可以如下所示:
```c
// 创建一个新的链表
ListNode* createCircularList() {
ListNode* head = NULL;
ListNode* tail = NULL;
if (head == NULL) {
head = (ListNode*)malloc(sizeof(ListNode)); // 分配内存给头节点
if (head != NULL) {
head->data = 0; // 初始化头节点数据
head->next = head; // 设置头节点的 next 和 tail 为自身
head->tail = head;
} else {
printf("Memory allocation failed.\n");
return NULL;
}
}
return head;
}
// 插入元素到链表的特定位置
void insertAtEnd(ListNode** head, int value) {
ListNode* newNode = (ListNode*)malloc(sizeof(ListNode));
if (newNode != NULL) {
newNode->data = value;
newNode->next = *head;
newNode->tail = *head->tail;
(*head)->tail->next = newNode;
*head->tail = newNode;
} else {
printf("Memory allocation for new node failed.\n");
}
}
// 删除指定值的节点(这里假设有头指针)
void deleteElement(ListNode** head, int value) {
ListNode* current = *head;
ListNode* prev = NULL;
while (current != NULL && current->data != value) {
prev = current;
current = current->next;
}
if (current != NULL) {
if (prev == NULL) { // 如果要删除的是头节点
*head = current->next;
if (*head != NULL) {
(*head)->tail = *head;
} else {
free(current); // 空链表时释放内存
}
} else {
prev->next = current->next;
current->tail->next = current;
free(current);
}
}
}
```
阅读全文
相关推荐
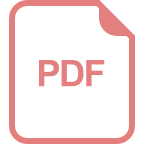
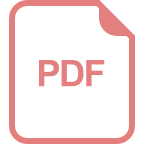
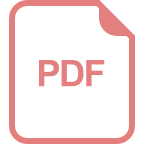
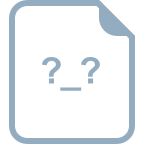




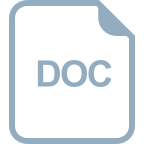









