c语言建立单循环链表
时间: 2023-08-24 09:07:20 浏览: 97
你可以使用以下代码来建立一个单循环链表的数据结构:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构
struct Node {
int data;
struct Node* next;
};
// 在链表末尾插入节点
void insertAtEnd(struct Node** head, int data) {
// 创建新节点
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
newNode->data = data;
newNode->next = NULL;
if (*head == NULL) {
// 如果链表为空,将新节点设为头节点
*head = newNode;
newNode->next = *head;
} else {
// 找到链表的尾节点
struct Node* tail = *head;
while (tail->next != *head) {
tail = tail->next;
}
// 将新节点插入到尾节点后面
tail->next = newNode;
newNode->next = *head;
}
}
// 遍历并打印链表中的元素
void printList(struct Node* head) {
if (head == NULL) {
printf("链表为空。\n");
return;
}
struct Node* current = head;
printf("链表的元素:");
do {
printf("%d ", current->data);
current = current->next;
} while (current != head);
printf("\n");
}
int main() {
struct Node* head = NULL; // 初始化头节点为空
// 在链表末尾插入节点
insertAtEnd(&head, 1);
insertAtEnd(&head, 2);
insertAtEnd(&head, 3);
insertAtEnd(&head, 4);
// 打印链表中的元素
printList(head);
return 0;
}
```
这段代码定义了一个 `Node` 结构来表示链表的节点,包含一个整数数据和一个指向下一个节点的指针。`insertAtEnd` 函数用于在链表末尾插入节点,`printList` 函数用于遍历并打印链表中的元素。在 `main` 函数中,我们创建一个头节点,并在链表末尾插入一些节点,然后打印链表中的元素。请注意,在插入第一个节点时,需要将新节点的 `next` 指针指向头节点,以形成循环链表。
阅读全文
相关推荐
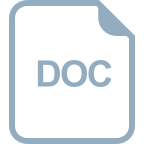
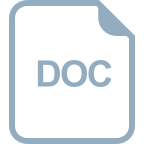
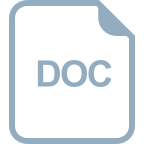


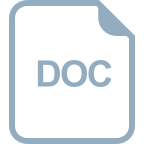
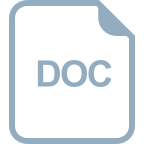
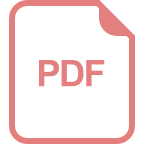







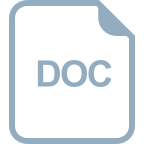
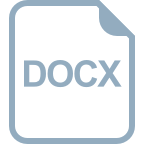