这段代码什么意思 std::string data(buffer, bytesRead);
时间: 2024-04-18 12:28:58 浏览: 106
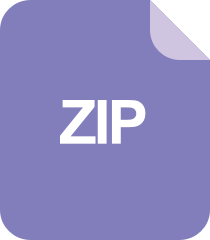
读取串口数据,java写的
`std::string data(buffer, bytesRead);` 这段代码的意思是使用从串口读取的数据 `buffer` 构造一个 `std::string` 对象 `data`。
在C++中,`std::string` 是一个字符串类,可以用于存储和处理文本数据。它提供了多种构造函数,其中之一接受字符数组和长度作为参数,用于从字符数组中构造字符串对象。
在这个代码段中,`buffer` 是一个字符数组,存储了从串口读取的数据,而 `bytesRead` 则表示读取到的数据字节数。通过使用 `std::string` 的构造函数,我们可以将 `buffer` 中的数据转换为字符串,并存储在 `data` 对象中。
这样,我们就可以方便地使用 `data` 对象来处理从串口读取的数据,例如进行字符串操作、解析或显示等。
阅读全文
相关推荐
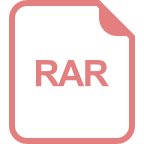
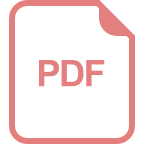
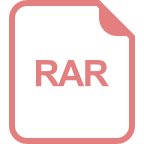
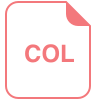














