python文本词性频率
时间: 2024-09-14 20:03:45 浏览: 35
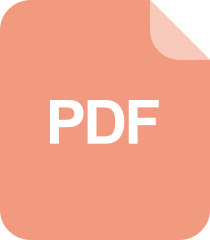
Python文本特征抽取与向量化算法学习
在Python中,文本词性(Part-of-Speech,POS)频率指的是对文本中的每个单词进行词性标注,并统计每种词性的出现次数。这通常涉及到自然语言处理(NLP)技术,其中的一个关键步骤就是使用词性标注器(Part-of-Speech Tagger)。
以下是一个简单的Python示例,展示如何使用`nltk`库来进行词性标注并统计词性频率:
```python
import nltk
from nltk.tokenize import word_tokenize
from collections import Counter
from nltk.corpus import wordnet
# 确保已经下载nltk的punkt tokenizer和averaged_perceptron_tagger
nltk.download('punkt')
nltk.download('averaged_perceptron_tagger')
# 示例文本
text = "Natural language processing (NLP) is a field of computer science, artificial intelligence, and linguistics concerned with the interactions between computers and human (natural) languages."
# 分词
words = word_tokenize(text)
# 进行词性标注
tagged = nltk.pos_tag(words)
# 将词性标注结果转换为更通用的词性标记(例如:'NN' -> 'n', 'VB' -> 'v')
def get_wordnet_pos(treebank_tag):
if treebank_tag.startswith('J'):
return wordnet.ADJ
elif treebank_tag.startswith('V'):
return wordnet.VERB
elif treebank_tag.startswith('N'):
return wordnet.NOUN
elif treebank_tag.startswith('R'):
return wordnet.ADV
else:
return None
# 使用转换后的词性标记
tagged = [(word, get_wordnet_pos(pos) or 'n') for word, pos in tagged]
# 统计词性频率
tag_counts = Counter(tag for word, tag in tagged)
# 打印词性频率
for tag, count in tag_counts.items():
print(f"词性 {tag}: {count}次")
# 输出词性频率
```
上述代码段做了以下几步操作:
1. 对一段示例文本进行分词处理。
2. 使用`nltk`的词性标注器对分词后的结果进行标注。
3. 将标注结果中的词性标签转换为`wordnet`的词性标记格式。
4. 使用`Counter`统计每种词性的出现次数。
5. 输出每种词性的名称和对应的频率。
请注意,`nltk`中的词性标注器返回的是基于Penn Treebank的词性标记,这里通过一个转换函数将其转换为`wordnet`的标记格式,以便于分析。
阅读全文
相关推荐
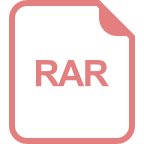
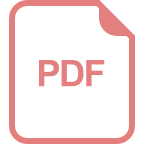
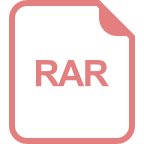
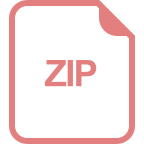
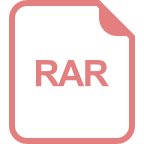
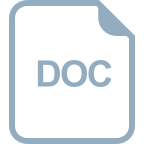
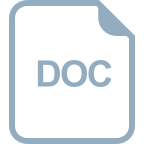
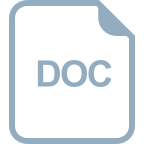
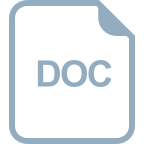
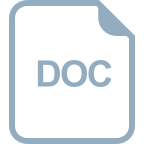
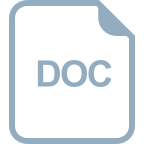
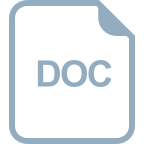
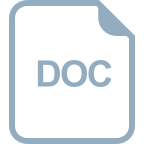
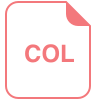



