如何计算从特定年份和月份的第一天到指定日期之间的天数?c语言
时间: 2024-12-24 10:28:18 浏览: 5
在C语言中,你可以通过一些基本的数学运算和时间处理函数来计算两个日期之间相隔的天数。以下是一个简单的步骤:
1. 首先,你需要获取给定年份和月份第一天的日期。这通常涉及到创建一个结构体来表示日期,比如`struct date`,包含年、月、日三个成员。
```c
typedef struct {
int year;
int month;
int day;
} Date;
```
2. 然后定义一个函数来获取特定月份的第一天。例如,闰年的二月有29天,其他月份有固定的天数。
```c
Date get_first_day_of_month(int year, int month) {
Date first_day;
first_day.year = year;
first_day.month = month;
switch (month) {
case 1: // January
first_day.day = 1;
break;
case 2: // February
if ((year % 4 == 0 && year % 100 != 0) || (year % 400 == 0)) {
first_day.day = 29; // Leap year
} else {
first_day.day = 28;
}
break;
// ... handle the rest of the months
}
return first_day;
}
```
3. 接下来,定义一个函数来计算两个日期之间的差值,可以是直接相减,然后取绝对值得到天数。
```c
int days_between_dates(Date start_date, Date end_date) {
int start_days_in_year = start_date.year * 365 + start_date.month <= 2 ? start_date.month - 1 : start_date.month;
int end_days_in_year = end_date.year * 365 + end_date.month - 1;
// Account for leap years and days in each month
return abs(end_days_in_year - start_days_in_year + (end_date.day - start_date.day));
}
```
请注意,这个方法非常基础,并未考虑实际的闰秒和复杂情况。对于精确的日期计算,建议使用更专业的库,如`<time.h>`或第三方日期库。
阅读全文
相关推荐
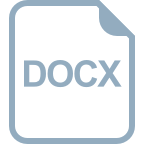
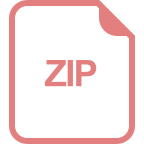
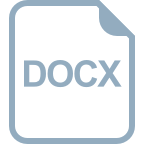
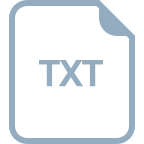
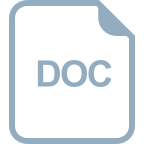
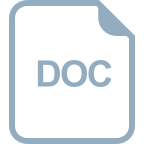
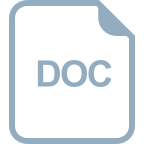
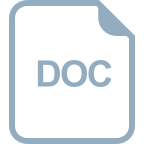
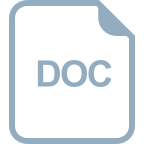
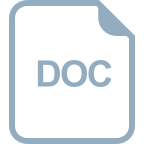
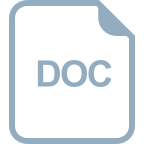
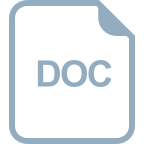
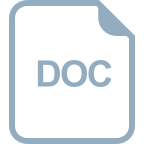





