编写一个工厂模式的样例,假设基类为Shape类,派生类为Cirdle(圆)和Rectangular (形),方法为GetShapeName, 要求过基类对象调用派生类的方法
时间: 2023-07-16 16:13:13 浏览: 60
下面是一个工厂模式的示例,其中基类为Shape类,派生类为Circle(圆)和Rectangular(矩形),方法为GetShapeName。
```c++
#include <iostream>
#include <string>
using namespace std;
// 基类 Shape
class Shape {
public:
virtual string GetShapeName() = 0;
virtual ~Shape() {}
};
// 派生类 Circle
class Circle : public Shape {
public:
string GetShapeName() override {
return "Circle";
}
};
// 派生类 Rectangular
class Rectangular : public Shape {
public:
string GetShapeName() override {
return "Rectangular";
}
};
// 工厂类 ShapeFactory
class ShapeFactory {
public:
static Shape* CreateShape(string type) {
if (type == "Circle") {
return new Circle();
} else if (type == "Rectangular") {
return new Rectangular();
}
return nullptr;
}
};
int main() {
Shape* shape1 = ShapeFactory::CreateShape("Circle");
Shape* shape2 = ShapeFactory::CreateShape("Rectangular");
cout << shape1->GetShapeName() << endl; // 输出:Circle
cout << shape2->GetShapeName() << endl; // 输出:Rectangular
delete shape1;
delete shape2;
return 0;
}
```
在上面的示例中,我们定义了基类 Shape 和两个派生类 Circle 和 Rectangular。工厂类 ShapeFactory 可以创建 Circle 和 Rectangular 对象。我们可以使用基类 Shape 的指针来调用派生类的 GetShapeName() 方法。
相关推荐
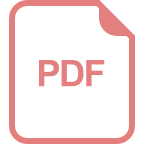
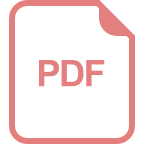














