编写一个Shell程序,检查用户对HelloWorld内核模块是否有执行权限,如果没有则赋予其执行权限,加载该内核模块,每隔10s检查一次该内核模块是否已经正确加载
时间: 2023-06-13 22:07:50 浏览: 52
以下是一个可能的Shell程序示例:
```sh
#!/bin/bash
# 检查用户是否有执行权限
if ! test -x HelloWorld.ko; then
echo "没有执行权限,赋予执行权限"
chmod +x HelloWorld.ko
fi
# 加载内核模块
echo "加载内核模块"
insmod HelloWorld.ko
# 每隔10s检查一次内核模块是否已正确加载
while true; do
if lsmod | grep HelloWorld > /dev/null; then
echo "内核模块已正确加载"
break
else
echo "内核模块未正确加载,等待10s"
sleep 10
fi
done
```
这个程序的思路是首先检查用户是否有执行权限,如果没有则赋予其执行权限,然后加载内核模块。接着进入一个循环,每隔10秒钟检查一次内核模块是否已经正确加载,如果已经加载则退出循环,否则继续等待。在循环中,使用lsmod命令来列出已加载的内核模块,并使用grep命令来查找HelloWorld模块,如果找到则表示已正确加载。注意,这个程序可能需要使用root权限才能正常运行。
相关问题
编写内核模块,使用tasklet实现打印helloworld。
首先,我们需要创建一个基本的内核模块,可以参考以下代码:
```c
#include <linux/init.h>
#include <linux/module.h>
#include <linux/kernel.h>
#include <linux/interrupt.h>
MODULE_LICENSE("GPL");
MODULE_AUTHOR("Your Name");
MODULE_DESCRIPTION("Tasklet Example");
static unsigned long tasklet_data = 0;
// Tasklet function
static void hello_tasklet(unsigned long data)
{
printk(KERN_INFO "Hello, world! (tasklet)\n");
}
// Declare tasklet
DECLARE_TASKLET(my_tasklet, hello_tasklet, tasklet_data);
// Module initialization function
static int __init tasklet_init(void)
{
printk(KERN_INFO "Tasklet Example: Initializing\n");
// Schedule tasklet
tasklet_schedule(&my_tasklet);
return 0;
}
// Module exit function
static void __exit tasklet_exit(void)
{
printk(KERN_INFO "Tasklet Example: Exiting\n");
// Disable and free tasklet
tasklet_disable(&my_tasklet);
tasklet_kill(&my_tasklet);
}
// Register module initialization and exit functions
module_init(tasklet_init);
module_exit(tasklet_exit);
```
在上面的代码中,我们使用了 `DECLARE_TASKLET` 宏来声明一个名为 `my_tasklet` 的 tasklet,它执行的函数是 `hello_tasklet`。在 `tasklet_init` 中,我们使用 `tasklet_schedule` 函数来调度 tasklet 的执行。在 `tasklet_exit` 中,我们使用 `tasklet_disable` 和 `tasklet_kill` 函数来禁用和释放 tasklet。
编译和加载内核模块:
```shell
make
sudo insmod tasklet_example.ko
```
查看内核日志:
```shell
dmesg
```
输出应该类似于以下内容:
```
[ 163.123456] Tasklet Example: Initializing
[ 163.123456] Hello, world! (tasklet)
[ 163.123456] Tasklet Example: Exiting
```
注意,输出中的时间戳可能会发生变化。
使用Linux中的moudule模块的编程基本方法,并可以将其动态加载入内核,举个例子
好的,下面是一个简单的例子,展示如何使用Linux中的模块进行编程,并将其动态加载到内核中。
我们假设要编写一个简单的内核模块,用于在内核启动时输出一些信息。具体步骤如下:
1. 创建一个新的目录,用于存放模块的源代码和Makefile文件。
2. 创建模块的源代码文件,如test.c文件,内容如下:
```
#include <linux/module.h>
#include <linux/kernel.h>
int init_module(void)
{
printk(KERN_INFO "Hello, world!\n");
return 0;
}
void cleanup_module(void)
{
printk(KERN_INFO "Goodbye, world!\n");
}
```
3. 创建Makefile文件,内容如下:
```
obj-m += test.o
KERNELDIR ?= /lib/modules/$(shell uname -r)/build
PWD := $(shell pwd)
default:
$(MAKE) -C $(KERNELDIR) M=$(PWD) modules
clean:
$(MAKE) -C $(KERNELDIR) M=$(PWD) clean
```
4. 编译模块代码,使用命令“make”进行编译。
5. 使用insmod命令将模块加载到内核中,如“insmod test.ko”命令。
6. 在系统日志中查看模块的输出信息,如“dmesg | tail”命令。
7. 使用rmmod命令将模块从内核中卸载,如“rmmod test”命令。
这个例子中,我们定义了一个简单的模块,包括初始化函数和清理函数。在初始化函数中,我们使用printk函数输出一条信息。在清理函数中,我们同样使用printk函数输出一条信息。编译模块代码后,我们可以使用insmod命令将模块加载到内核中,在系统日志中查看模块的输出信息。使用rmmod命令将模块从内核中卸载。
相关推荐
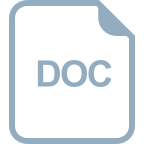
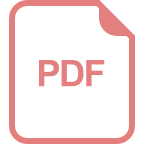
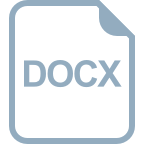












