#include <osg/BezierCurves>对应的cpp文件源码
时间: 2024-06-08 13:06:26 浏览: 139
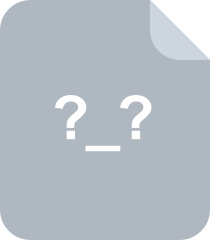
源代码cpp
很抱歉,这个问题的回答需要更多的上下文信息。
`osg/BezierCurves` 是 OpenSceneGraph 库中用于实现贝塞尔曲线的头文件之一,但是它并不是一个完整的类或函数,而是一个包含了一些函数声明和类声明的头文件。因此,如果您想要查看 `osg/BezierCurves` 中的具体实现,需要先了解调用了这个头文件的代码所在的上下文以及使用的具体函数或类。
如果您能提供更多的上下文信息或者代码片段,我可以更准确地回答您的问题。
阅读全文
相关推荐
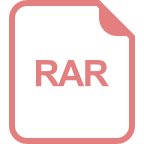


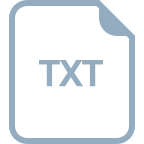
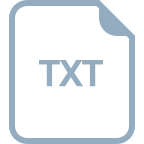
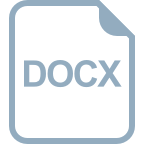
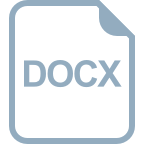



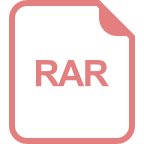
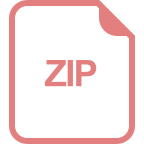
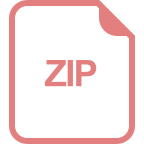
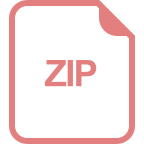