// 从文件中加载参赛队伍信息 void loadTeamsFromFile(vector<Team>& teams) { ifstream inFile("team.txt"); if (!inFile.is_open()) { cout << "无法打开team.txt文件!" << endl; return; } string line; while (getline(inFile, line)) { stringstream ss(line); Team team; if (ss >> team.id) { ss >> std::ws; getline(ss, team.name, '\t'); ss >> std::ws; getline(ss, team.school, '\t'); ss >> std::ws; getline(ss, team.category, '\t'); ss >> std::ws; getline(ss, team.players, '\t'); ss >> std::ws; getline(ss, team.coach); cout << "id: " << team.id << ", name: " << team.name << ", school: " << team.school << ", category: " << team.category << ", players: " << team.players << ", coach: " << team.coach << endl; teams.push_back(team); } else { cout << "无效行:" << line << endl; } } } 根据这段代码写出之后按学校查找的代码
时间: 2024-01-22 16:03:51 浏览: 66
以下是按学校查找的代码示例:
``` c++
// 按学校查找参赛队伍
void searchTeamsBySchool(const vector<Team>& teams, const string& school) {
vector<Team> result; // 保存查找结果
for (const auto& team : teams) {
if (team.school == school) {
result.push_back(team);
}
}
if (result.empty()) {
cout << "没有找到 " << school << " 的参赛队伍。" << endl;
}
else {
cout << school << " 的参赛队伍如下:" << endl;
for (const auto& team : result) {
cout << "id: " << team.id << ", name: " << team.name << ", school: " << team.school
<< ", category: " << team.category << ", players: " << team.players << ", coach: " << team.coach << endl;
}
}
}
```
你可以将该函数添加到原有代码中,并在需要查找时调用该函数。
阅读全文
相关推荐
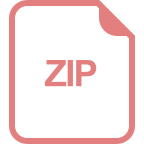
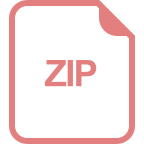
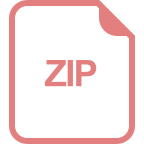

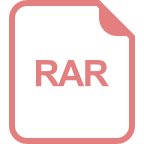
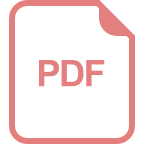
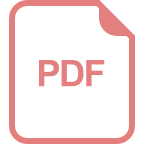
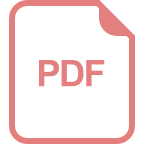
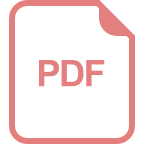
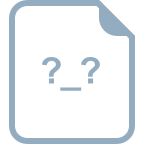
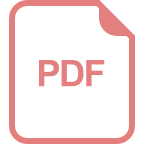
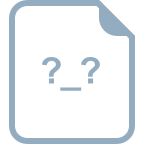
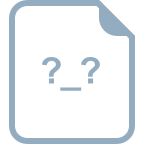
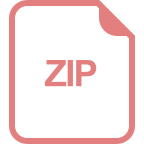
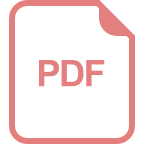
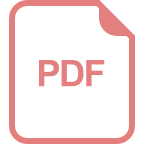