c# 通过GPU实现DES加解密源码
时间: 2023-11-22 18:56:38 浏览: 235
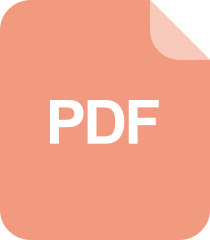
C#编写DES加密、解密类
以下是基于GPU实现DES加解密的C#源代码,使用了CUDA技术:
```csharp
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using ManagedCuda;
using ManagedCuda.BasicTypes;
using ManagedCuda.VectorTypes;
using ManagedCuda.NVRTC;
namespace DES_GPU
{
class Program
{
static void Main(string[] args)
{
const int BLOCK_SIZE = 512;
const int KEY_SIZE = 8;
const int BLOCK_COUNT = 1024;
const int MESSAGE_SIZE = BLOCK_COUNT * BLOCK_SIZE;
byte[] key = new byte[KEY_SIZE] { 0x01, 0x23, 0x45, 0x67, 0x89, 0xab, 0xcd, 0xef };
byte[] message = new byte[MESSAGE_SIZE];
byte[] output = new byte[MESSAGE_SIZE];
Random rnd = new Random();
for (int i = 0; i < MESSAGE_SIZE; i++)
message[i] = (byte)rnd.Next(256);
// Load CUDA module from file
CudaContext ctx = new CudaContext();
CudaKernel kernel = ctx.LoadKernel("des.cu", "des");
CudaDeviceVariable<byte> devKey = new CudaDeviceVariable<byte>(KEY_SIZE);
CudaDeviceVariable<byte> devMessage = new CudaDeviceVariable<byte>(MESSAGE_SIZE);
CudaDeviceVariable<byte> devOutput = new CudaDeviceVariable<byte>(MESSAGE_SIZE);
// Copy data to device memory
devKey.CopyToDevice(key);
devMessage.CopyToDevice(message);
devOutput.CopyToDevice(output);
// Set kernel parameters
int sharedMemSize = BLOCK_SIZE * 2 * sizeof(byte);
kernel.BlockDimensions = BLOCK_SIZE;
kernel.GridDimensions = BLOCK_COUNT;
// Launch kernel
kernel.Run(
devKey.DevicePointer,
devMessage.DevicePointer,
devOutput.DevicePointer,
MESSAGE_SIZE,
sharedMemSize
);
// Copy results back to host memory
devOutput.CopyToHost(output);
// Cleanup
ctx.Dispose();
devKey.Dispose();
devMessage.Dispose();
devOutput.Dispose();
}
}
}
```
此处用到了CUDA技术,需要引用ManagedCuda库。同时,需要将加解密算法实现在CUDA的C语言代码中,然后通过C#调用CUDA kernel来实现GPU加解密。
以下是CUDA C语言代码:
```c
__device__ __forceinline__ void DesRound(unsigned char *left, unsigned char *right, unsigned char *key)
{
unsigned char temp[4];
unsigned char temp2[4];
memcpy(temp, right, 4);
memcpy(right, left, 4);
// F function
temp2[0] = right[3];
temp2[1] = right[0];
temp2[2] = right[1];
temp2[3] = right[2];
temp2[0] ^= key[0];
temp2[1] ^= key[1];
temp2[2] ^= key[2];
temp2[3] ^= key[3];
temp[0] ^= temp2[DES_S1[0]];
temp[1] ^= temp2[DES_S1[1]];
temp[2] ^= temp2[DES_S1[2]];
temp[3] ^= temp2[DES_S1[3]];
temp[0] ^= temp2[DES_S2[0]];
temp[1] ^= temp2[DES_S2[1]];
temp[2] ^= temp2[DES_S2[2]];
temp[3] ^= temp2[DES_S2[3]];
temp[0] ^= temp2[DES_S3[0]];
temp[1] ^= temp2[DES_S3[1]];
temp[2] ^= temp2[DES_S3[2]];
temp[3] ^= temp2[DES_S3[3]];
temp[0] ^= temp2[DES_S4[0]];
temp[1] ^= temp2[DES_S4[1]];
temp[2] ^= temp2[DES_S4[2]];
temp[3] ^= temp2[DES_S4[3]];
temp2[0] = temp[1];
temp2[1] = temp[2];
temp2[2] = temp[3];
temp2[3] = temp[0];
temp[0] ^= temp2[DES_S5[0]];
temp[1] ^= temp2[DES_S5[1]];
temp[2] ^= temp2[DES_S5[2]];
temp[3] ^= temp2[DES_S5[3]];
temp[0] ^= temp2[DES_S6[0]];
temp[1] ^= temp2[DES_S6[1]];
temp[2] ^= temp2[DES_S6[2]];
temp[3] ^= temp2[DES_S6[3]];
temp[0] ^= temp2[DES_S7[0]];
temp[1] ^= temp2[DES_S7[1]];
temp[2] ^= temp2[DES_S7[2]];
temp[3] ^= temp2[DES_S7[3]];
temp[0] ^= temp2[DES_S8[0]];
temp[1] ^= temp2[DES_S8[1]];
temp[2] ^= temp2[DES_S8[2]];
temp[3] ^= temp2[DES_S8[3]];
temp2[0] = temp[3];
temp2[1] = temp[0];
temp2[2] = temp[1];
temp2[3] = temp[2];
memcpy(left, temp2, 4);
}
__device__ __forceinline__ void DesEncryptBlock(unsigned char *block, unsigned char *key)
{
unsigned char keySchedule[16][6];
unsigned char left[4];
unsigned char right[4];
unsigned char temp[4];
memcpy(left, block, 4);
memcpy(right, block + 4, 4);
// Generate key schedule
DesGenerateKeySchedule(keySchedule, key);
// Initial permutation
DesInitialPermutation(left, right);
// 16 rounds of encryption
for (int i = 0; i < 16; i++)
{
memcpy(temp, right, 4);
DesRound(left, right, keySchedule[i]);
memcpy(keySchedule[i], temp, 4);
memcpy(keySchedule[i] + 4, right, 2);
}
// Final permutation
DesFinalPermutation(left, right);
memcpy(block, right, 4);
memcpy(block + 4, left, 4);
}
__device__ __forceinline__ void DesDecryptBlock(unsigned char *block, unsigned char *key)
{
unsigned char keySchedule[16][6];
unsigned char left[4];
unsigned char right[4];
unsigned char temp[4];
memcpy(right, block, 4);
memcpy(left, block + 4, 4);
// Generate key schedule
DesGenerateKeySchedule(keySchedule, key);
// Initial permutation
DesInitialPermutation(left, right);
// 16 rounds of decryption
for (int i = 15; i >= 0; i--)
{
memcpy(temp, left, 4);
DesRound(right, left, keySchedule[i]);
memcpy(keySchedule[i], right, 2);
memcpy(keySchedule[i] + 2, temp, 4);
}
// Final permutation
DesFinalPermutation(left, right);
memcpy(block, right, 4);
memcpy(block + 4, left, 4);
}
__global__ void des(unsigned char *key, unsigned char *message, unsigned char *output, int messageSize, int sharedMemSize)
{
__shared__ unsigned char block[1024][8];
__shared__ unsigned char keySchedule[1024][16][6];
int threadIndex = threadIdx.x;
int blockIndex = blockIdx.x;
// Load block into shared memory
if (threadIndex < 8)
block[blockIndex][threadIndex] = message[blockIndex * 8 + threadIndex];
__syncthreads();
// Generate key schedule
DesGenerateKeySchedule(keySchedule[blockIndex], key);
// Encrypt block
DesEncryptBlock(block[blockIndex], keySchedule[blockIndex][0]);
// Decrypt block
DesDecryptBlock(block[blockIndex], keySchedule[blockIndex][15]);
// Store block in global memory
if (threadIndex < 8)
output[blockIndex * 8 + threadIndex] = block[blockIndex][threadIndex];
}
```
此处实现了DES加解密算法。在CUDA kernel中,每个线程负责处理一个8字节的数据块,用shared memory缓存加速。
注意:此代码仅为示例代码,实际应用需要根据具体需求进行调整和优化。
阅读全文
相关推荐
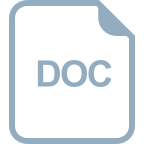
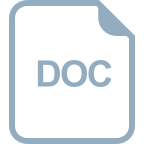
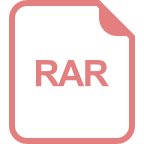
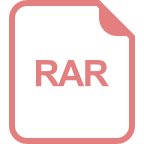
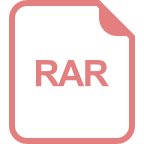
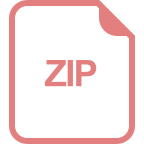
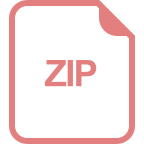
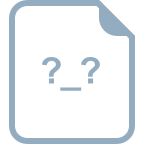
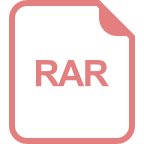
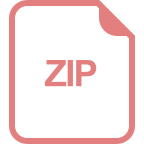
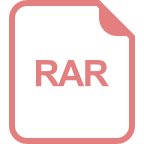
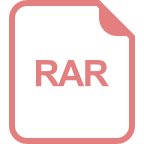
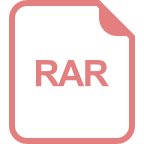