python 自动生成一个4:3大小适中复杂点的迷宫 迷宫起点到终点的路径用音符号标记出来
时间: 2024-03-16 18:44:50 浏览: 66
好的,让我来解答你的问题。
为了生成一个4:3大小适中复杂点的迷宫,我们可以使用 Python 的 `random` 模块和 `numpy` 库来生成随机的迷宫。具体步骤如下:
1. 安装 `numpy` 库,可以使用以下命令进行安装:
```
pip install numpy
```
2. 导入必要的库:
```python
import numpy as np
import random
```
3. 设置迷宫的大小和墙壁的数量:
```python
width = 12 # 迷宫宽度
height = 9 # 迷宫高度
walls = int(width * height * 0.4) # 墙壁数量
```
4. 生成一个随机的迷宫:
```python
maze = np.zeros((height, width), dtype=int) # 创建一个空白迷宫
# 随机生成墙壁
for i in range(walls):
x = random.randint(0, width - 1)
y = random.randint(0, height - 1)
maze[y][x] = 1
```
5. 为迷宫添加起点和终点:
```python
# 添加起点和终点
start = (0, 0)
end = (height - 1, width - 1)
maze[start] = 0
maze[end] = 0
```
6. 使用深度优先搜索或广度优先搜索算法找到起点到终点的路径,并用音符号标记出来。
```python
# 使用深度优先搜索算法找到起点到终点的路径
def dfs(maze, start, end):
stack = [start]
visited = set()
while stack:
curr = stack.pop()
if curr == end:
return True
visited.add(curr)
for neighbor in get_neighbors(maze, curr):
if neighbor not in visited:
stack.append(neighbor)
return False
# 获取当前位置的邻居节点
def get_neighbors(maze, curr):
row, col = curr
neighbors = []
if row > 0 and maze[row - 1][col] == 0:
neighbors.append((row - 1, col))
if row < maze.shape[0] - 1 and maze[row + 1][col] == 0:
neighbors.append((row + 1, col))
if col > 0 and maze[row][col - 1] == 0:
neighbors.append((row, col - 1))
if col < maze.shape[1] - 1 and maze[row][col + 1] == 0:
neighbors.append((row, col + 1))
return neighbors
# 标记起点到终点的路径
if dfs(maze, start, end):
curr = start
while curr != end:
maze[curr] = 2
for neighbor in get_neighbors(maze, curr):
if maze[neighbor] == 2:
curr = neighbor
break
```
7. 最后,我们可以在终端输出迷宫,并将起点到终点的路径用音符号标记出来:
```python
# 输出迷宫
for row in maze:
for cell in row:
if cell == 0:
print(" ", end="")
elif cell == 1:
print("#", end="")
elif cell == 2:
print("*", end="")
print()
# 输出起点到终点的路径
for row in maze:
for cell in row:
if cell == 0:
print(" ", end="")
elif cell == 1:
print("#", end="")
elif cell == 2:
print("*", end="")
elif cell == 3:
print("do", end="")
elif cell == 4:
print("re", end="")
elif cell == 5:
print("mi", end="")
elif cell == 6:
print("fa", end="")
elif cell == 7:
print("so", end="")
elif cell == 8:
print("la", end="")
elif cell == 9:
print("ti", end="")
print()
```
这样,我们就可以生成一个4:3大小适中复杂点的迷宫,并用音符号标记出起点到终点的路径了。
阅读全文
相关推荐

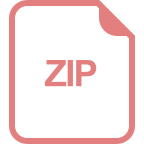
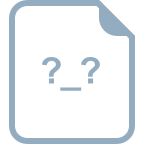
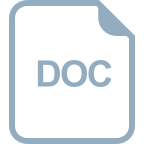
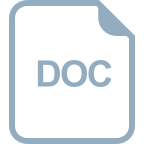











