我现在有一个文件夹A,A 文件夹中有多个子文件夹,我现在需要将文件夹A 以及子文件夹中的所有表格文件的信息,汇总到一个新表格中。将该新表格命名为 666,并将它存到桌面上
时间: 2024-10-19 10:14:10 浏览: 15
为了完成这个任务,你可以按照以下步骤操作:
1. **打开程序**:首先,你需要使用一个支持处理文件操作的软件,例如Microsoft Excel、Google Sheets或Python的数据处理库如pandas。
2. **遍历文件夹**:如果你是在Windows系统下,可以使用Python的`os`模块通过递归函数遍历整个文件夹A及其子文件夹,查找所有的表格文件(通常扩展名为`.xls`, `.xlsx`, 或`.csv`等)。
```python
import os
from pandas import read_excel
def find_files(start_path):
excel_files = []
for root, dirs, files in os.walk(start_path):
for file in files:
if file.endswith(('.xls', '.xlsx', '.csv')):
excel_files.append(os.path.join(root, file))
return excel_files
excel_list = find_files('文件夹A')
```
3. **读取数据**:然后对每个找到的表格文件使用相应的库读取内容,例如`read_excel`对于Excel文件。
```python
data_frames = []
for file in excel_list:
df = read_excel(file)
data_frames.append(df)
```
4. **合并数据**:把所有子文件夹里的表格数据合并成一个大表,如果它们有相同的列名,可以使用`pd.concat`或者`pandas.DataFrame.append`。
```python
combined_df = pd.concat(data_frames, ignore_index=True) # 或者 combined_df = pd.DataFrame()
for df in data_frames:
combined_df = combined_df.append(df, ignore_index=True)
```
5. **保存新表格**:最后,将合并后的`combined_df`存储为新的CSV或Excel文件(假设命名为"666.xlsx"),并保存到桌面。
```python
new_file_path = os.path.join(os.environ['USERPROFILE'], 'Desktop', '666.xlsx') # 获取桌面路径
combined_df.to_excel(new_file_path, index=False)
```
6. **确认结果**:运行以上脚本后,你应该会在桌面上看到名为"666.xlsx"的新表格文件,包含了所有子文件夹中的表格数据。
**相关问题--:**
1. 如果遇到权限问题,如何解决?
2. 如果表格文件非常大,如何优化内存使用?
3. 怎么在Mac系统上做类似的操作?
阅读全文
相关推荐
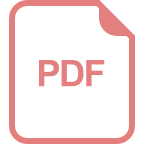
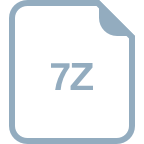
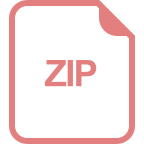

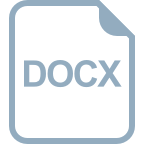
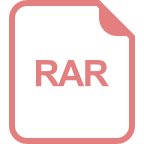
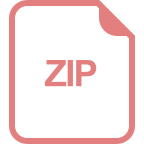
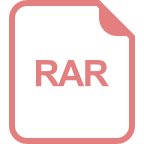
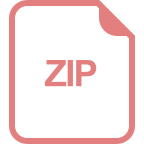
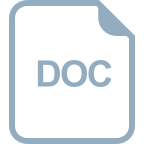
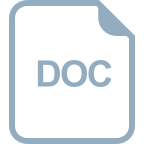
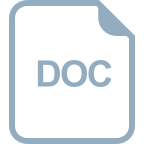
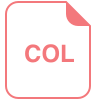
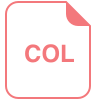




