c++中string的find用法
时间: 2023-12-01 15:03:07 浏览: 36
在C语言中,没有字符串类型和find函数,不过可以通过使用标准库中的字符串处理函数来实现类似的功能。
可以使用strchr函数来查找字符串中某个字符第一次出现的位置,例如:
```c
char str[] = "hello world";
char *ptr = strchr(str, 'w');
if (ptr != NULL) {
printf("字符 'w' 在字符串中第一次出现的位置是:%d\n", ptr - str);
} else {
printf("字符 'w' 在字符串中未找到\n");
}
```
另外,可以使用strstr函数来查找字符串中一个子串第一次出现的位置,例如:
```c
char str[] = "hello world";
char *ptr = strstr(str, "world");
if (ptr != NULL) {
printf("子串 'world' 在字符串中第一次出现的位置是:%d\n", ptr - str);
} else {
printf("子串 'world' 在字符串中未找到\n");
}
```
需要注意的是,这些函数在找到匹配的位置时返回的是指向该位置的指针,如果未找到则返回NULL。此外,指针相减的结果是两个指针之间的距离,也就是偏移量。
相关问题
c++中string.find()函数用法
在C++中,string.find()函数用于在一个字符串中查找另一个字符串的位置。其语法如下:
```c++
size_t find (const string& str, size_t pos = 0) const noexcept;
```
其中,第一个参数是要查找的字符串,第二个参数是从哪个位置开始查找(默认值为0)。
该函数返回查找到的第一个字符的位置,如果未找到,则返回string::npos。
下面是一个示例:
```c++
#include <iostream>
#include <string>
using namespace std;
int main() {
string str = "Hello, world!";
size_t found = str.find("world");
if (found != string::npos) {
cout << "Found at position " << found << endl;
} else {
cout << "Not found" << endl;
}
return 0;
}
```
输出结果为:
```
Found at position 7
```
c++中string 的用法
以下是C++中string的常用用法:
1. 定义和初始化string变量
```c++
string str1; // 定义一个空字符串
string str2 = "hello"; // 定义并初始化一个字符串
string str3("world"); // 使用构造函数定义并初始化一个字符串
```
2. 字符串的拼接
```c++
string str4 = str2 + " " + str3; // 使用+运算符拼接字符串
str1.append(str2); // 使用append()函数将str2连接到str1的结尾
str1.append(str2, 2, 3); // 将str2从第2个字符开始的3个字符连接到str1的结尾
```
3. 字符串的查找和替换
```c++
int pos = str4.find("world"); // 查找子串"world"在str4中的位置
str4.replace(pos, 5, "there"); // 将str4中从pos开始的5个字符替换为"there"
int rpos = str4.rfind("e"); // 查找字符'e'在str4中最后一次出现的位置
```
4. 字符串的截取和转换
```c++
string str5 = str4.substr(6, 5); // 截取str4中从第6个字符开始的5个字符
const char* cstr = str5.c_str(); // 将string类型的str5转换为C风格的字符串
int num = stoi(str2); // 将字符串str2转换为整数类型
double dnum = stod(str3); // 将字符串str3转换为双精度浮点数类型
```
相关推荐
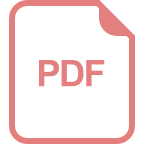
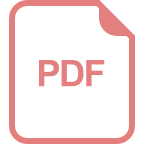
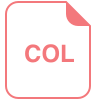
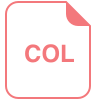
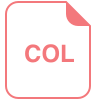
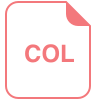
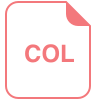







