用Python 写一个基于遗传算法的排班代码
时间: 2024-02-27 18:59:21 浏览: 22
好的,以下是基于遗传算法的排班代码示例:
```python
import random
# 定义员工信息和班次信息
employees = ["A", "B", "C", "D", "E", "F", "G", "H"]
shifts = ["早班", "中班", "晚班"]
# 定义遗传算法参数
POPULATION_SIZE = 20
GENERATIONS = 100
MUTATION_RATE = 0.1
# 定义适应度函数,计算并返回适应度值
def fitness(individual):
score = 0
for i in range(len(individual)):
if individual[i] == shifts[0]:
if employees[i] not in ["B", "E", "G"]:
score += 1
elif individual[i] == shifts[1]:
if employees[i] not in ["A", "C", "D", "F"]:
score += 1
else:
if employees[i] not in ["A", "C", "E", "H"]:
score += 1
return score
# 定义交叉函数,返回两个父代的子代
def crossover(parent1, parent2):
crossover_point = random.randint(1, len(parent1)-1)
child1 = parent1[:crossover_point] + parent2[crossover_point:]
child2 = parent2[:crossover_point] + parent1[crossover_point:]
return child1, child2
# 定义变异函数,返回变异后的个体
def mutation(individual):
mutated_individual = individual[:]
gene_to_mutate = random.randint(0, len(mutated_individual)-1)
mutated_individual[gene_to_mutate] = random.choice(shifts)
return mutated_individual
# 初始化种群
population = []
for i in range(POPULATION_SIZE):
individual = [random.choice(shifts) for j in range(len(employees))]
population.append(individual)
# 迭代
for generation in range(GENERATIONS):
# 计算适应度值
fitness_scores = []
for individual in population:
fitness_scores.append(fitness(individual))
# 找到适应度值最高的个体
best_individual_index = fitness_scores.index(max(fitness_scores))
best_individual = population[best_individual_index]
print("第{}代最优解:{},适应度值:{}".format(generation, best_individual, max(fitness_scores)))
# 选择
selected_parents = []
for i in range(2):
parent1 = population[random.randint(0, POPULATION_SIZE-1)]
parent2 = population[random.randint(0, POPULATION_SIZE-1)]
if fitness(parent1) > fitness(parent2):
selected_parents.append(parent1)
else:
selected_parents.append(parent2)
# 交叉
child1, child2 = crossover(selected_parents[0], selected_parents[1])
# 变异
if random.random() < MUTATION_RATE:
child1 = mutation(child1)
if random.random() < MUTATION_RATE:
child2 = mutation(child2)
# 更新种群
population[best_individual_index] = child1
population[random.randint(0, POPULATION_SIZE-1)] = child2
```
这是一个简单的排班程序,使用遗传算法来生成最优的员工排班方案。程序中,我们定义了员工信息和班次信息,以及遗传算法的参数。适应度函数根据员工能否胜任某个班次来计算适应度值,交叉和变异函数用来生成新的个体。在迭代过程中,我们选择适应度值最高的两个个体作为父代,通过交叉和变异生成两个子代,并更新种群。最终,程序会输出每一代的最优解和适应度值。
相关推荐
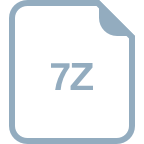














