请你给我写一个简单的遗传算法的Python代码
时间: 2024-04-14 13:28:01 浏览: 150
当然,下面是一个简单的遗传算法的 Python 代码示例:
```python
import numpy as np
POP_SIZE = 50 # 种群大小
GENE_LENGTH = 10 # 染色体长度
CROSS_RATE = 0.8 # 交叉概率
MUTATION_RATE = 0.01 # 变异概率
N_GENERATIONS = 100 # 迭代次数
# 初始化种群
population = np.random.randint(0, 2, (POP_SIZE, GENE_LENGTH))
# 定义适应度函数
def fitness(population):
return np.sum(population, axis=1)
# 定义选择操作
def select(population, fitness):
idx = np.random.choice(np.arange(POP_SIZE), size=POP_SIZE, replace=True, p=fitness/fitness.sum())
return population[idx]
# 定义交叉操作
def crossover(parents):
offspring = np.empty((POP_SIZE, GENE_LENGTH))
for i in range(POP_SIZE):
p1, p2 = parents[i], parents[np.random.randint(0, POP_SIZE)]
crossover_point = np.random.randint(0, 2, GENE_LENGTH).astype(np.bool)
offspring[i] = p1
offspring[i, crossover_point] = p2[crossover_point]
return offspring
# 定义变异操作
def mutate(offspring):
for i in range(POP_SIZE):
for j in range(GENE_LENGTH):
if np.random.rand() < MUTATION_RATE:
offspring[i, j] = 1 - offspring[i, j]
return offspring
# 主循环
for generation in range(N_GENERATIONS):
# 计算适应度
fit = fitness(population)
# 选择操作
parents = select(population, fit)
# 交叉操作
offspring = crossover(parents)
# 变异操作
offspring = mutate(offspring)
# 更新种群
population = offspring
# 打印最优个体
best_idx = np.argmax(fit)
print("Best individual:", population[best_idx])
```
这个示例代码实现了一个简单的遗传算法,通过随机生成二进制的染色体,并进行选择、交叉和变异操作,最终找到适应度最高的个体作为最优解。遗传算法的核心思想是通过模拟生物进化的过程,在种群中搜索最优解。
阅读全文
相关推荐
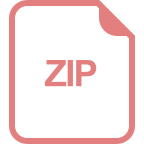
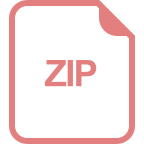















