你能帮我写一个遗传算法的代码吗?
时间: 2023-05-28 18:02:51 浏览: 82
当然可以!但是遗传算法的具体实现需要根据具体问题来设计,以下是一个简单的遗传算法示例,你可以根据自己的需求进行修改和扩展。
遗传算法实现步骤:
1. 生成初始种群(population);
2. 计算每个个体(individual)的适应度(fitness)值;
3. 选择(selection):根据适应度值选择优秀的个体;
4. 交叉(crossover):通过交叉操作产生新的个体;
5. 变异(mutation):对个体进行变异操作以增加多样性;
6. 重复步骤2到步骤5,直到达到指定条件。
下面是一个遗传算法的 Python 代码示例,实现了一个简单的函数优化问题。
```python
import random
# 定义目标函数:f(x) = x**2
def target_function(x):
return x**2
# 计算适应度值
def fitness_func(x):
return 1 / (target_function(x) + 1)
# 生成初始种群
def generate_population(size, lower_bound, upper_bound):
population = []
for i in range(size):
individual = random.uniform(lower_bound, upper_bound)
population.append(individual)
return population
# 选择函数(采用轮盘赌选择算法)
def roulette_selection(population, fitness):
total_fitness = sum(fitness)
pick = random.uniform(0, total_fitness)
current = 0
for i in range(len(population)):
current += fitness[i]
if current > pick:
return population[i]
# 交叉函数
def crossover(parent1, parent2):
# 选择交叉点
crossover_point = random.randint(1, len(parent1) - 1)
# 交叉
child1 = parent1[:crossover_point] + parent2[crossover_point:]
child2 = parent2[:crossover_point] + parent1[crossover_point:]
return child1, child2
# 变异函数
def mutation(individual, lower_bound, upper_bound):
# 选择变异位置
mutation_point = random.randint(0, len(individual) - 1)
# 变异(加入随机扰动)
individual = list(individual)
individual[mutation_point] += random.uniform(-0.1, 0.1)
# 超出边界的处理
if individual[mutation_point] > upper_bound:
individual[mutation_point] = upper_bound
elif individual[mutation_point] < lower_bound:
individual[mutation_point] = lower_bound
return ''.join(individual)
# 遗传算法主体函数
def genetic_algorithm(target_func, size, lower_bound, upper_bound, max_iter):
# 初始化种群
population = generate_population(size, lower_bound, upper_bound)
# 计算初始适应度值
fitness = [fitness_func(x) for x in population]
# 迭代
for i in range(max_iter):
# 选择
parent1 = roulette_selection(population, fitness)
parent2 = roulette_selection(population, fitness)
# 交叉
child1, child2 = crossover(parent1, parent2)
# 变异
child1 = mutation(child1, lower_bound, upper_bound)
child2 = mutation(child2, lower_bound, upper_bound)
# 替换
population[random.randint(0, size-1)] = child1
population[random.randint(0, size-1)] = child2
# 计算适应度值
fitness = [fitness_func(x) for x in population]
# 返回最优解
best_individual = population[fitness.index(max(fitness))]
return best_individual
# 测试
best_x = genetic_algorithm(target_function, 50, -10, 10, 100)
print('best x:', best_x)
print('best f(x):', target_function(best_x))
```
上面这个示例是一个简单的实现,只是优化了一个函数,如果更复杂的问题需要复杂的算法实现,希望我的回答对你有帮助!
相关推荐
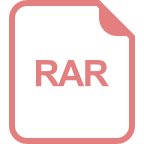
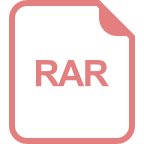
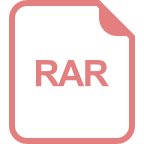














