建立一个表示结点的模板类,类中包括一个任意类型的数据和一个结点指针。在主函数中使用该模板类建立数据类型为整型的单向链表,并把链表中的所有数据打印出来
时间: 2024-05-02 17:23:51 浏览: 10
好的,您的问题是关于如何建立一个表示结点的模板类,类中包括一个任意类型的数据和一个结点指针。在主函数中使用该模板类建立数据类型为整型的单向链表,并把链表中的所有数据打印出来。下面是一个可能的实现:
```
#include <iostream>
using namespace std;
template<class T>
class Node {
public:
T data;
Node<T>* next;
Node(T value) {
data = value;
next = NULL;
}
};
template<class T>
class LinkedList {
public:
Node<T>* head;
LinkedList() {
head = NULL;
}
void add(T value) {
Node<T>* newNode = new Node<T>(value);
if (head == NULL) {
head = newNode;
}
else {
Node<T>* current = head;
while (current->next != NULL) {
current = current->next;
}
current->next = newNode;
}
}
void printList() {
Node<T>* current = head;
while (current != NULL) {
cout << current->data << " ";
current = current->next;
}
cout << endl;
}
};
int main() {
LinkedList<int> list;
list.add(1);
list.add(2);
list.add(3);
list.add(4);
list.add(5);
list.printList();
return 0;
}
```
在这个实现中,我们使用了一个表示结点的模板类 `Node`,其中包括一个任意类型的数据和一个结点指针。我们还定义了一个单向链表的模板类 `LinkedList`,其中包括一个头指针和添加和打印链表的方法。
在主函数中,我们建立了数据类型为整型的单向链表,并添加了一些数据。最后,我们打印了链表中的所有数据。
相关推荐
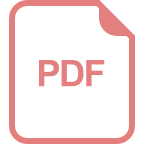
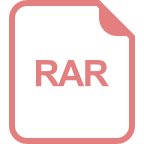















