从深度图获取点云python
时间: 2023-10-02 07:07:55 浏览: 94
可以使用Open3D库中的函数来从深度图获取点云。以下是一个简单的示例代码:
```python
import numpy as np
import open3d as o3d
# 加载深度图
depth_image = o3d.io.read_image("depth.png")
# 摄像机内参
fx = 500 # 焦距x
fy = 500 # 焦距y
cx = 320 # 光心x
cy = 240 # 光心y
# 生成点云
intrinsics = o3d.camera.PinholeCameraIntrinsic(width=depth_image.width, height=depth_image.height, fx=fx, fy=fy, cx=cx, cy=cy)
depth = o3d.geometry.Image(depth_image)
rgbd = o3d.geometry.RGBDImage.create_from_depth_image(depth, intrinsics)
pcd = o3d.geometry.PointCloud.create_from_rgbd_image(rgbd, intrinsics)
# 可视化点云
o3d.visualization.draw_geometries([pcd])
```
在上面的代码中,首先读取深度图文件,并定义了摄像机内参。然后使用Open3D库中的函数生成点云,并通过可视化函数将其可视化。
相关问题
深度图 点云 python
深度图和点云是计算机视觉中常用的两种数据表示形式,常用于场景重建、三维感知和物体识别等任务。
深度图是一种灰度图像,其中每个像素的值表示该像素与相机的距离,通常以灰度级或可视化调色板的形式呈现。深度图可以通过各种方法获取,例如激光测距、双目视觉和飞行时间等。
点云是一种三维数据表示形式,由一组三维点坐标组成,每个点表示场景中的一个物体或表面的一个样本。点云可以通过各种传感器获得,如激光雷达、深度摄像机和结构光等。
Python是一种广泛使用的编程语言,具有丰富的科学计算和计算机视觉库,如NumPy、OpenCV和PCL(点云库)。Python可以用于处理和分析深度图和点云数据,进行各种应用和算法的开发。
在Python中,可以使用OpenCV库来读取和处理深度图像。可以通过使用cv2模块提供的函数来读取、显示和保存深度图像,并根据像素值提取物体边界或进行图像分割。
对于点云数据的处理,可以使用NumPy库来表示和处理点云数据。点云通常以二维数组的形式表示,其中每一行表示一个三维点的坐标。可以通过使用NumPy提供的功能来处理点云数据,例如平移、旋转、滤波和配准等操作。
此外,还可以使用PCL库来进行更高级的点云处理和算法。PCL是一个功能强大的点云处理库,可以用于点云配准、分割、分类和特征提取等任务。在Python中可以通过pybind11或者PCL接口来调用PCL库。
综上所述,深度图和点云是计算机视觉中常用的两种数据表示形式,Python是一种方便易用的编程语言,可以用于处理和分析这些数据。
用奥比中光深度相机获取点云的python代码
以下是使用奥比中光深度相机获取点云的Python代码示例:
```python
import openni2
import numpy as np
# 初始化奥比中光深度相机
openni2.initialize()
# 打开设备
dev = openni2.Device.open_any()
# 创建深度流
depth_stream = dev.create_depth_stream()
# 启动深度流
depth_stream.start()
# 获取深度图像
depth_frame = depth_stream.read_frame()
# 将深度图像转换为numpy数组
depth_array = np.array(depth_frame.get_buffer_as_uint16())
# 获取相机参数
depth_stream_profile = depth_stream.get_video_stream().get_sensor_info().get_stream_profile()
intrinsics = depth_stream_profile.get_intrinsics()
# 计算点云
fx = intrinsics.fx
fy = intrinsics.fy
cx = intrinsics.ppx
cy = intrinsics.ppy
depth_scale = depth_stream.get_depth_scale()
depth_array = depth_array.astype(float) * depth_scale
rows, cols = depth_array.shape
c, r = np.meshgrid(np.arange(cols), np.arange(rows), sparse=True)
valid = depth_array > 0
z = np.where(valid, depth_array, np.nan)
x = np.where(valid, z * (c - cx) / fx, 0)
y = np.where(valid, z * (r - cy) / fy, 0)
point_cloud = np.dstack((x, y, z))
# 关闭深度流
depth_stream.stop()
depth_stream.destroy()
# 关闭设备
dev.close()
# 反初始化奥比中光深度相机
openni2.unload()
```
以上代码中,首先使用`openni2.initialize()`初始化奥比中光深度相机,然后使用`dev.create_depth_stream()`创建深度流,并使用`depth_stream.start()`启动深度流。接着,使用`depth_stream.read_frame()`获取深度图像,并使用`depth_frame.get_buffer_as_uint16()`将深度图像转换为numpy数组。然后,使用`depth_stream.get_video_stream().get_sensor_info().get_stream_profile()`获取相机参数,并计算点云。最后,使用`depth_stream.stop()`关闭深度流,使用`dev.close()`关闭设备,最后使用`openni2.unload()`反初始化奥比中光深度相机。
阅读全文
相关推荐
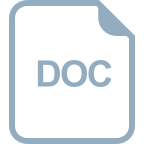
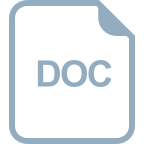
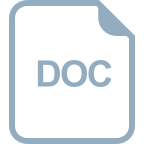
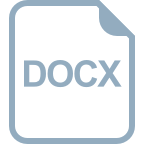









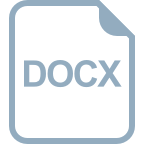


