使用命令模式设计一个图形绘制系统,该系统可以绘制矩形、椭圆、三角形等图形,恢复和撤销功能。绘制类图并编程实现。java实现
时间: 2024-06-08 16:05:36 浏览: 38
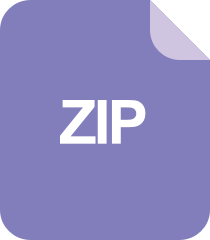
基于java语言开发的图形设计、图像绘制信息管理系统的毕业设计实现.zip
好的,下面是命令模式的类图:

在这个类图中,我们可以看到以下几个角色:
- `Command`: 命令接口,定义了执行和撤销命令的方法。
- `ConcreteCommand`: 具体命令类,实现了命令接口,包含了一个对于接收者的引用,用于执行具体的操作。
- `Receiver`: 接收者,真正执行命令的对象。
- `Invoker`: 调用者,用于调用命令并管理命令的历史记录。
- `Client`: 客户端,负责创建命令对象并将其传递给调用者。
下面是一份基于该类图的 Java 代码实现:
```java
// Command.java
public interface Command {
void execute();
void undo();
}
// RectangleCommand.java
public class RectangleCommand implements Command {
private Receiver receiver;
private int x1, y1, x2, y2;
public RectangleCommand(Receiver receiver, int x1, int y1, int x2, int y2) {
this.receiver = receiver;
this.x1 = x1;
this.y1 = y1;
this.x2 = x2;
this.y2 = y2;
}
@Override
public void execute() {
receiver.drawRectangle(x1, y1, x2, y2);
}
@Override
public void undo() {
receiver.eraseRectangle(x1, y1, x2, y2);
}
}
// EllipseCommand.java
public class EllipseCommand implements Command {
private Receiver receiver;
private int x1, y1, x2, y2;
public EllipseCommand(Receiver receiver, int x1, int y1, int x2, int y2) {
this.receiver = receiver;
this.x1 = x1;
this.y1 = y1;
this.x2 = x2;
this.y2 = y2;
}
@Override
public void execute() {
receiver.drawEllipse(x1, y1, x2, y2);
}
@Override
public void undo() {
receiver.eraseEllipse(x1, y1, x2, y2);
}
}
// TriangleCommand.java
public class TriangleCommand implements Command {
private Receiver receiver;
private int x1, y1, x2, y2, x3, y3;
public TriangleCommand(Receiver receiver, int x1, int y1, int x2, int y2, int x3, int y3) {
this.receiver = receiver;
this.x1 = x1;
this.y1 = y1;
this.x2 = x2;
this.y2 = y2;
this.x3 = x3;
this.y3 = y3;
}
@Override
public void execute() {
receiver.drawTriangle(x1, y1, x2, y2, x3, y3);
}
@Override
public void undo() {
receiver.eraseTriangle(x1, y1, x2, y2, x3, y3);
}
}
// Receiver.java
public class Receiver {
public void drawRectangle(int x1, int y1, int x2, int y2) {
// 绘制矩形
}
public void eraseRectangle(int x1, int y1, int x2, int y2) {
// 擦除矩形
}
public void drawEllipse(int x1, int y1, int x2, int y2) {
// 绘制椭圆
}
public void eraseEllipse(int x1, int y1, int x2, int y2) {
// 擦除椭圆
}
public void drawTriangle(int x1, int y1, int x2, int y2, int x3, int y3) {
// 绘制三角形
}
public void eraseTriangle(int x1, int y1, int x2, int y2, int x3, int y3) {
// 擦除三角形
}
}
// Invoker.java
import java.util.Stack;
public class Invoker {
private Stack<Command> undoStack = new Stack<>();
private Stack<Command> redoStack = new Stack<>();
public void execute(Command command) {
command.execute();
undoStack.push(command);
redoStack.clear();
}
public void undo() {
if (!undoStack.isEmpty()) {
Command command = undoStack.pop();
command.undo();
redoStack.push(command);
}
}
public void redo() {
if (!redoStack.isEmpty()) {
Command command = redoStack.pop();
command.execute();
undoStack.push(command);
}
}
}
// Client.java
public class Client {
public static void main(String[] args) {
Receiver receiver = new Receiver();
Invoker invoker = new Invoker();
// 创建命令对象
Command command1 = new RectangleCommand(receiver, 10, 10, 100, 100);
Command command2 = new EllipseCommand(receiver, 20, 20, 200, 100);
Command command3 = new TriangleCommand(receiver, 30, 30, 150, 150, 200, 50);
// 执行命令
invoker.execute(command1);
invoker.execute(command2);
invoker.execute(command3);
// 撤销命令
invoker.undo();
invoker.undo();
// 重做命令
invoker.redo();
}
}
```
在这份代码中,我们使用了 `Receiver` 来表示真正执行命令的对象,`Invoker` 用于调用命令并管理命令的历史记录,而 `Client` 则负责创建命令对象并将其传递给 `Invoker`。可以看到,每个具体命令类都包含了一个对于接收者的引用,这个引用在执行命令时被使用。同时,每个具体命令类也实现了 `undo()` 方法,用于撤销该命令所做的操作。
阅读全文
相关推荐






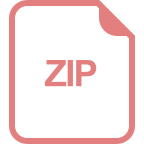
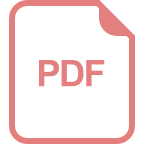
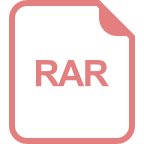
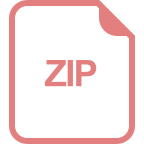
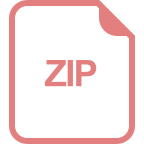
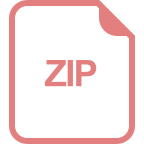
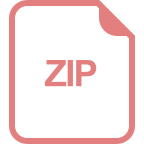