while not leave: python
时间: 2023-10-14 22:57:27 浏览: 96
在Python中,`while`循环用于重复执行一段代码直到满足某个条件时才停止。在你提供的代码中,`while not leave:`表示当`leave`变量为`False`时,循环将一直执行。你可以在循环体内编写你想要重复执行的代码。当满足某个条件时,你可以将`leave`变量设置为`True`,以退出循环。
相关问题
queue python
A queue in Python is a linear data structure that follows the First-In-First-Out (FIFO) principle. It is similar to a real-life queue, where the first person to enter the queue is the first person to leave it.
In Python, a queue can be implemented using the built-in module 'queue'. There are two types of queues that can be created using this module: the 'Queue' class and the 'PriorityQueue' class.
The 'Queue' class is a basic queue that follows the FIFO principle. It has the following methods:
- put(item): Adds an item to the end of the queue.
- get(): Removes and returns the item at the front of the queue.
- empty(): Returns True if the queue is empty, False otherwise.
- qsize(): Returns the number of items in the queue.
The 'PriorityQueue' class is a queue that orders the items based on their priority. It has the same methods as the 'Queue' class, but it also has a 'put(item, priority)' method that allows the user to specify the priority of the item being added.
Here's an example of how to create and use a 'Queue' object in Python:
```
import queue
q = queue.Queue()
q.put(1)
q.put(2)
q.put(3)
while not q.empty():
print(q.get())
```
Output:
```
1
2
3
```
In this example, we create a 'Queue' object and add three items to it using the 'put' method. We then use a while loop to remove and print each item using the 'get' method. Since the queue follows the FIFO principle, the items are printed in the order they were added.
如何使用Python中的wx库实现一个功能:当用户鼠标悬停在窗口上的按钮上时,窗口会随机调整其位置?请提供相应的Python代码示例。
要在Python中使用wx库创建一个窗口并实现鼠标悬停时随机调整窗口位置的功能,你需要首先安装`wxpython`库,如果你还没有安装,可以使用pip安装:
```bash
pip install wxpython
```
然后,你可以使用以下代码示例来实现这个功能:
```python
import wx
import random
class HoverMoveWindow(wx.Frame):
def __init__(self):
super().__init__(None, title="Hover Move Window", size=(400, 300))
self.button = wx.Button(self, label="Click me!", pos=(50, 50))
self.Bind(wx.EVT_LEFT_DOWN, self.on_left_down)
self.Bind(wx.EVT_ENTER_WINDOW, self.on_enter_window)
self.Bind(wx.EVT_LEAVE_WINDOW, self.on_leave_window)
def on_left_down(self, event):
# 用户点击事件,这里只是为了触发窗口移动,实际应用中可以处理其他逻辑
pass
def on_enter_window(self, event):
if event.LeftIsDown():
while True:
x, y = random.randint(0, self.GetClientSize().width), random.randint(0, self.GetClientSize().height)
if not self.Rect.Contains(x, y): # 避免窗口移出屏幕边界
break
self.SetPosition((x, y)) # 设置新的窗口位置
wx.Yield() # 更新界面
def on_leave_window(self, event):
# 当鼠标离开窗口时停止移动
self.StopMoving()
app = wx.App()
window = HoverMoveWindow()
window.Show(True)
app.MainLoop()
```
在这个例子中,我们创建了一个`HoverMoveWindow`类,当鼠标进入窗口并且左键按下时(实际上这里只做了一次左键按下,你可以修改成持续监听),窗口的位置会在窗口内部随机变化。当鼠标离开窗口时,窗口停止移动。
阅读全文
相关推荐
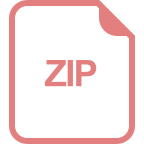
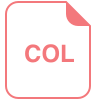
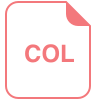
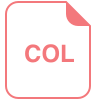
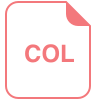
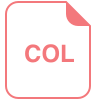
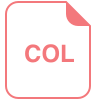







