Model Interpretability and Evaluation: Balancing Complexity with Interpretability
发布时间: 2024-09-15 14:24:51 阅读量: 26 订阅数: 23 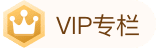
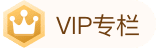
# 1. The Importance of Model Interpretability and Evaluation
In the realm of data science today, the performance of machine learning models is crucial, but so is their interpretability. Model interpretability refers to the ability to understand the reasons and processes behind a model's specific predictions or decisions. Its importance stems from several aspects:
- **Trust Building**: In critical application areas, such as healthcare and finance, the transparency of models can enhance trust among users and regulatory bodies.
- **Error Diagnosis**: Interpretability helps us identify and correct errors in the model, optimizing its performance.
- **Compliance Requirements**: Many industries have regulatory requirements that mandate the ability to explain the decision-making process of models to comply with legal stipulations.
To ensure model interpretability, it is necessary to establish and employ various evaluation methods and metrics to monitor and enhance model performance. These methods and metrics span every step from data preprocessing to model deployment, ensuring that while models pursue predictive accuracy, they also provide clear and understandable decision logic. In the following sections, we will delve into the theoretical foundations of model interpretability, different types of interpretation methods, and specific techniques for evaluating model performance.
# 2. Theoretical Foundations and Model Complexity
### 2.1 Theoretical Framework of Model Interpretability
#### 2.1.1 What is Model Interpretability
Model interpretability refers to the transparency and understandability of model predictions, that is, the ability to clearly explain to users how a model makes specific predictions. In the field of artificial intelligence, models are often viewed as "black boxes" because they typically contain complex parameters and structures that make it difficult for laypeople to understand their internal mechanisms. The importance of interpretability not only lies in increasing the transparency of the model but is also crucial for increasing user trust in model outcomes, diagnosing errors, and enhancing the reliability of the model.
#### 2.1.2 The Relationship Between Interpretability and Model Complexity
Model complexity is an important indicator for measuring a model's predictive power, learning efficiency, ***plex models, such as deep neural networks, excel at handling nonlinear problems but are difficult to understand internally, increasing their lack of interpretability. On the other hand, simpler models, such as linear regression models, are more intuitive but may perform inadequately when dealing with complex patterns. Ideally, models should maintain sufficient complexity to achieve the desired performance while also striving to improve their interpretability.
### 2.2 Measures of Model Complexity
#### 2.2.1 Time Complexity and Space Complexity
Time complexity and space complexity are two primary indicators for measuring the resource consumption of algorithms. Time complexity describes the trend of growth in the time required for an algorithm to execute as the input scale increases, commonly expressed using Big O notation. Space complexity is a measure of the amount of storage space an algorithm uses during execution. For machine learning models, time complexity is typically reflected in training and prediction times, while space complexity is evident in model size and storage requirements. When selecting models, in addition to considering model performance, it is also necessary to balance the constraints of time and space.
#### 2.2.2 Model Capacity and Generalization Ability
Model capacity refers to the ability of a model to capture complex patterns in data. High-capacity models (e.g., deep neural networks) can fit complex functions but are at high risk of overfitting, potentially performing poorly when generalizing to unknown data. The level of model capacity is determined not only by the model structure but also by the number of model parameters, the choice of activation functions, etc. Generalization ability refers to the model's predictive power for unseen examples. The complexity of the model needs to match its generalization ability to ensure that the model not only memorizes the training data but also learns the underlying patterns in the data.
### 2.3 The Relationship Between Complexity and Overfitting
#### 2.3.1 Causes and Consequences of Overfitting
Overfitting occurs when a model learns the training data too well, capturing noise and details that are not universally applicable in new, unseen data. Overfitting typically occurs when the model capacity is too high or when there is insufficient training data. The consequence is that the model performs well on the training set but significantly worse on validation or test sets. Overfitting not only affects the predictive accuracy of the model but also reduces its generalization ability, resulting in unreliable predictions when applied in practice.
#### 2.3.2 Strategies to Avoid Overfitting
There are various strategies to avoid overfitting, including but not limited to: increasing the amount of training data, data augmentation, reducing model complexity, introducing regularization terms, using cross-validation, and early stopping of training. These strategies help balance the learning and generalization abilities of the model to varying degrees. For instance, regularization techniques add a penalty term (e.g., L1, L2 regularization) to limit the size of model parameters, thus preventing the model from fitting too closely to the training data. These methods can improve the generalization ability of the model and reduce the risk of overfitting.
In the next chapter, we will delve deeper into interpretability methods and techniques and discuss how to apply these technologies to enhance the transparency and interpretability of models. We will first introduce local interpretability methods, such as LIME and SHAP, then move on to global interpretability methods, such as model simplification and rule-based interpretation frameworks. Finally, we will discuss model visualization techniques and how these technologies help us understand the working principles of models more intuitively.
# 3. Interpretability Methods and Techniques
## 3.1 Local Interpretability Methods
### 3.1.1 Principles and Applications of LIME and SHAP
Local Interpretable Model-agnostic Explanations (LIME) and SHapley Additive exPlanations (SHAP) are two popular local interpretability methods that help understand a model's behavior on specific instances by providing a succinct explanation for each prediction.
The core idea of LIME is to approximate the predictive behavior of the original model within the local space of an instance. It learns a simplified model that captures the behavior of the original model in that local by perturbing the input data and observing the changes in output. It is applicable to any model, including tabular and image data.
```python
from lime import LimeTabularExplainer
from sklearn.datasets import load_iris
from sklearn.ensemble import RandomForestClassifier
# Load dataset
data = load_iris()
X, y = data.data, data.target
# Train a random forest model as the black box model
model = RandomForestClassifier()
model.fit(X, y)
# Create a LIME explainer
explainer = LimeTabularExplainer(X, feature_names=data.feature_names, class_names=data.target_names)
# Select a data point for explanation
idx = 10
exp = explainer.explain_instance(X[idx], model.predict_proba, num_features=4)
exp.show_in_notebook(show_table=True, show_all=False)
```
In the code above, we first load the Iris dataset and train a random forest classifier. Then we create a `LimeTabularExplainer` instance and use it to explain the prediction results of the 11th sample in the dataset.
SHAP is a method based on game theory that uses the average marginal contribution of the feature value function to explain predictions. SHAP values assign a value to each feature, indicating the contribution of that feature to the prediction result.
```python
import shap
import numpy as np
# Use SHAP's TreeExplainer, designed for tree models
explainer = shap.TreeExplainer(model)
shap_values = explainer.shap_values(X)
# Visualize the SHAP values for the first prediction
shap.initjs()
shap.force_plot(explainer.expected_value[0], shap_values[0][idx,:], X[idx,:])
```
In this code snippet, we use the `TreeExplainer` to calculate the SHAP values for each sample and then use the `force_plot` method to generate an interactive visualization chart that shows the contribution of the model to the specific sample's prediction result.
### 3.1.2 Feature Importance Assessment Techniques
Feature importance is a core concept in model interpretability that helps us understand which features play a key role in model predictions. There are various methods to assess feature importance, including model-specific methods (such as feature importance from random forests) and model-agnostic methods (such as permutation importance).
```python
import eli5
from sklearn.ensemble import RandomForestClassifier
# Use the eli5 library to compute feature importance
perm = eli5.permutation_importance(model, X, y, n_iter=100)
eli5.show_weights(perm, feature_names=data.feature_names, show_stdv=True)
```
Here, we use the `eli5` library's `permutation_importance` function to compute the permutation importance of the model and use the `sho
0
0
相关推荐
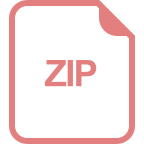
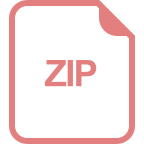
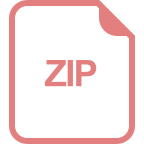
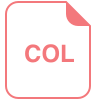
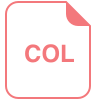
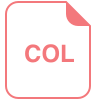
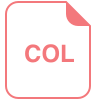
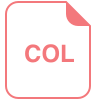
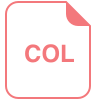