3.编写类模版,实现顺序栈的功能: (1)栈的初始化 (2)判断栈是否为空; (3)判断栈是否为满; (4)入栈; (5)出栈; (6)销毁。 并写出调用此类模板的完整程序。
时间: 2024-02-17 15:03:24 浏览: 73
以下是类模板实现顺序栈的代码:
```c++
#include<iostream>
using namespace std;
template <typename T>
class SeqStack {
public:
SeqStack(int n = 10);
~SeqStack();
bool isEmpty() const;
bool isFull() const;
bool push(const T& x);
bool pop(T& x);
void clear();
private:
T *data;
int top, maxSize;
};
template <typename T>
SeqStack<T>::SeqStack(int n) {
data = new T[n];
top = -1;
maxSize = n;
}
template <typename T>
SeqStack<T>::~SeqStack() {
delete[] data;
}
template <typename T>
bool SeqStack<T>::isEmpty() const {
return top == -1;
}
template <typename T>
bool SeqStack<T>::isFull() const {
return top == maxSize - 1;
}
template <typename T>
bool SeqStack<T>::push(const T& x) {
if (isFull()) {
return false;
}
data[++top] = x;
return true;
}
template <typename T>
bool SeqStack<T>::pop(T& x) {
if (isEmpty()) {
return false;
}
x = data[top--];
return true;
}
template <typename T>
void SeqStack<T>::clear() {
top = -1;
}
int main() {
SeqStack<int> stack(5);
cout << "stack is empty: " << stack.isEmpty() << endl;
cout << "push 1: " << stack.push(1) << endl;
cout << "push 2: " << stack.push(2) << endl;
cout << "push 3: " << stack.push(3) << endl;
cout << "push 4: " << stack.push(4) << endl;
cout << "push 5: " << stack.push(5) << endl;
cout << "push 6: " << stack.push(6) << endl;
int x;
cout << "pop: " << stack.pop(x) << " value: " << x << endl;
cout << "pop: " << stack.pop(x) << " value: " << x << endl;
cout << "pop: " << stack.pop(x) << " value: " << x << endl;
cout << "pop: " << stack.pop(x) << " value: " << x << endl;
cout << "pop: " << stack.pop(x) << " value: " << x << endl;
cout << "stack is empty: " << stack.isEmpty() << endl;
return 0;
}
```
在主函数中,我们首先创建了一个存放 int 类型的顺序栈,然后对其进行了一系列的操作,包括检查是否为空、入栈、出栈等。
阅读全文
相关推荐
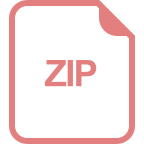
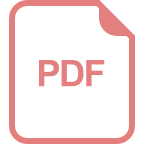
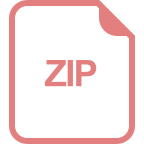











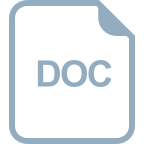
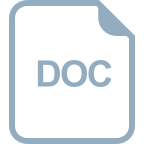
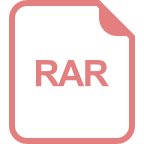
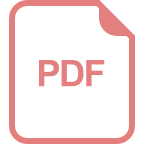