react 查询的请求 response 里显示 com.zbeats.commons.util.ResourceUtil$JsonResponse@12264f8d
时间: 2024-05-13 15:16:23 浏览: 14
这个问题可能是因为在接收到响应后,未将响应转换为 JSON 格式。你可以尝试使用 `response.json()` 方法将响应转换为 JSON 格式。例如:
```javascript
fetch(url)
.then(response => response.json())
.then(data => {
console.log(data); // 这里打印的是 JSON 格式的数据
})
.catch(error => console.error(error));
```
如果你的后端返回的响应不是 JSON 格式,你需要检查后端代码,确保返回的响应是 JSON 格式。
相关问题
eact 查询的请求 response 里显示 com.zbeats.commons.util.ResourceUtil$JsonResponse@12264f8d 解决示例代码
根据你提供的信息,我猜测你使用了 `ResourceUtil` 类来获取 JSON 数据,并将其转换为字符串以供 React 组件使用。但是,你在响应中看到了 `com.zbeats.commons.util.ResourceUtil$JsonResponse@12264f8d`,这是因为你没有正确地将 JSON 字符串返回给 React 组件。下面是一个可能的解决方案:
1. 确保在 ResourceUtil 类中正确地读取 JSON 数据。例如,你可以使用以下代码:
```java
public static String getJsonString(String path) throws IOException {
ObjectMapper mapper = new ObjectMapper(); // 创建 ObjectMapper 实例
InputStream inputStream = ResourceUtil.class.getResourceAsStream(path); // 读取 JSON 文件
JsonNode jsonNode = mapper.readTree(inputStream); // 将 JSON 数据转换为 JsonNode 对象
return mapper.writeValueAsString(jsonNode); // 将 JsonNode 对象转换为字符串并返回
}
```
2. 在处理请求时,确保将 JSON 字符串正确地返回给 React 组件。例如,你可以使用以下代码:
```java
@RequestMapping("/data")
@ResponseBody
public String getData() throws IOException {
String jsonString = ResourceUtil.getJsonString("/data.json"); // 获取 JSON 数据
return jsonString; // 返回 JSON 字符串
}
```
这样,当 React 组件请求该 URL 时,将返回正确的 JSON 字符串,而不是 `com.zbeats.commons.util.ResourceUtil$JsonResponse@12264f8d`。
使用 react-native-svg@13.9.0实现水波纹扩散动画
好的,我可以为您提供一些参考步骤:
1. 安装 react-native-svg@13.9.0
```
npm install --save react-native-svg@13.9.0
```
2. 创建一个新的组件,例如 WaterRippleAnimation.js
```javascript
import React, { Component } from 'react';
import { View, Animated } from 'react-native';
import Svg, { Circle } from 'react-native-svg';
class WaterRippleAnimation extends Component {
state = {
circleScale: new Animated.Value(0),
};
componentDidMount() {
Animated.timing(this.state.circleScale, {
toValue: 1,
duration: 1000,
useNativeDriver: true,
}).start();
}
render() {
const { circleScale } = this.state;
return (
<View style={{ flex: 1 }}>
<Svg height="100%" width="100%">
<Circle
cx="50%"
cy="50%"
r={circleScale.interpolate({
inputRange: [0, 1],
outputRange: ['0%', '80%'],
})}
fill="blue"
opacity={circleScale.interpolate({
inputRange: [0, 1],
outputRange: [0.5, 0],
})}
/>
</Svg>
</View>
);
}
}
export default WaterRippleAnimation;
```
3. 在需要使用水波纹动画的地方,导入 WaterRippleAnimation 组件并使用
```javascript
import React, { Component } from 'react';
import { View } from 'react-native';
import WaterRippleAnimation from './WaterRippleAnimation';
class App extends Component {
render() {
return (
<View style={{ flex: 1 }}>
<WaterRippleAnimation />
</View>
);
}
}
export default App;
```
这样就可以实现一个简单的水波纹扩散动画了。您可以根据自己的需求进一步调整动画效果。
相关推荐
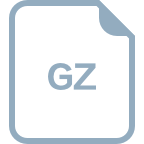
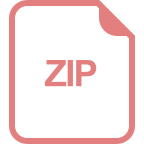












