用C++编写一个程序来计算矩形的面积。
时间: 2024-02-15 07:01:23 浏览: 180
一个简单的 C++ 程序可以使用以下代码来计算矩形的面积:
```
#include <iostream>
using namespace std;
int main() {
double width, height;
cout << "Enter the width of the rectangle: ";
cin >> width;
cout << "Enter the height of the rectangle: ";
cin >> height;
double area = width * height;
cout << "The area of the rectangle is: " << area << endl;
return 0;
}
```
这个程序先从用户处读取矩形的宽和高,然后计算矩形的面积并输出结果。
如果要编写一个面向对象的程序来计算矩形的面积,可以创建一个 Rectangle 类,其中包含成员变量 width 和 height,以及成员函数 getArea(),如下所示:
```
class Rectangle {
private:
double width;
double height;
public:
Rectangle(double w, double h) {
width = w;
height = h;
}
double getArea() {
return width * height;
}
};
```
然后在 main 函数中创建一个 Rectangle 对象,调用它的 getArea() 函数来计算矩形的面积,如下所示:
```
int main() {
double width, height;
cout << "Enter the width of the rectangle: ";
cin >> width;
cout << "Enter the height of the rectangle: ";
cin >> height;
Rectangle rect(width, height);
double area = rect.getArea();
cout << "The area of the rectangle is: " << area << endl;
return 0;
}
```
输出结果与之前的程序相同。
相关推荐
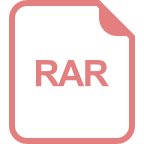














