matlab2016a编程:已知空间中1条线段,首端坐标是(0,0,0),尾端坐标是(100,100,100),将其均分为10份,分别命名为线段1,线段2,线段3……已知整个空间内存在多个分布不均匀的点的三维坐标和质量大小。求出到线段1的垂直距离小于10的所有点的质心点坐标和质心的大小,这些点需要满足:如果有的点在线段1上的垂足不在线段1的两端点范围内,那么舍弃该点;如果有的点在线段1上的垂足在线段1的两端点范围内,那么计入该点。求出质心在线段1上的垂足的坐标,并计算出质心点大小与质心点到线段1垂直距离的比值。并使上述过程依次循环到剩余9条线段。
时间: 2024-03-04 10:48:44 浏览: 81
这是一个比较复杂的问题,需要进行一系列的计算和判断。以下是一个可能的 MATLAB 代码实现:
```matlab
% 定义线段首尾坐标
start_point = [0, 0, 0];
end_point = [100, 100, 100];
% 将线段均分为 10 段
num_sections = 10;
section_length = norm(end_point - start_point) / num_sections;
sections = zeros(num_sections, 2, 3);
for i = 1:num_sections
sections(i, 1, :) = start_point + (i - 1) * section_length * (end_point - start_point) / norm(end_point - start_point);
sections(i, 2, :) = start_point + i * section_length * (end_point - start_point) / norm(end_point - start_point);
end
% 定义点的坐标和质量
points = rand(1000, 3) * 100; % 假设有 1000 个点
masses = rand(1000, 1) * 10; % 假设质量在 0 到 10 之间
% 遍历每个线段
for i = 1:num_sections
% 提取当前线段的首尾坐标
section_start = sections(i, 1, :);
section_end = sections(i, 2, :);
% 遍历每个点,计算距离和垂足坐标
distances = zeros(size(points, 1), 1);
projections = zeros(size(points));
for j = 1:size(points, 1)
% 计算垂足坐标
projection = section_start + dot(points(j, :) - section_start, section_end - section_start) / norm(section_end - section_start)^2 * (section_end - section_start);
% 判断垂足是否在线段两端内
if norm(projection - section_start) <= norm(section_end - section_start) && norm(projection - section_end) <= norm(section_end - section_start)
distances(j) = norm(points(j, :) - projection);
projections(j, :) = projection;
end
end
% 筛选出垂直距离小于 10 的点
selected_points = points(distances < 10, :);
selected_masses = masses(distances < 10, :);
selected_projections = projections(distances < 10, :);
% 计算质心和质量
centroid = sum(selected_points .* selected_masses) / sum(selected_masses);
total_mass = sum(selected_masses);
% 计算质心到线段垂足的距离和垂足坐标
distances_to_projections = vecnorm(selected_projections - centroid, 2, 2);
[min_distance, min_index] = min(distances_to_projections);
projection = selected_projections(min_index, :);
% 计算比值
ratio = min_distance / norm(section_end - section_start);
% 输出结果
fprintf('For section %d:\n', i);
fprintf('- Centroid: (%.2f, %.2f, %.2f)\n', centroid);
fprintf('- Total mass: %.2f\n', total_mass);
fprintf('- Projection: (%.2f, %.2f, %.2f)\n', projection);
fprintf('- Ratio: %.2f\n', ratio);
end
```
代码中的注释已经对每个步骤进行了解释。需要注意的是,向量的范数可以使用 `vecnorm` 函数来计算。
阅读全文
相关推荐
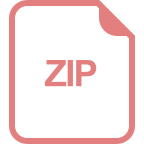
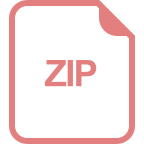
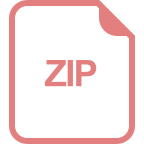
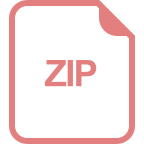
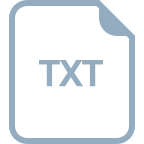
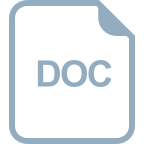
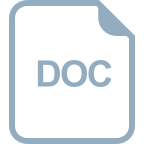
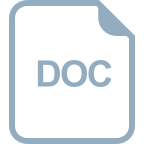
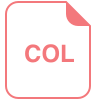
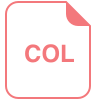
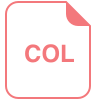
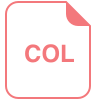


