设计一个图书类Book,数据成员有书号(id)、书名(bookTitle)、价格(price)、出版社(publisher);有带4个参数的构造函数,有静态数据成员图书对象总价bookTotalPrice;有静态函数getBookTotalPrice返回当前图书对象的总价格;getMessage()成员函数返回图书信息。编写测试程序,在main函数中输入若干图书信息,构建若干图书类对象,并测试类中的所有函数。提示:在函数中可以使用stringstream类实现构造所需字符串并返回字符串,例如: stringstream oss; oss<<""书号:""<<id<<"",书名:""<<bookTitle<<"",价格:""<<price<<"",出版社:""<<publisher; string message=oss.str(); return message; 注:stringstream类需要引入头文件#include<sstream>
时间: 2023-07-19 10:44:56 浏览: 87
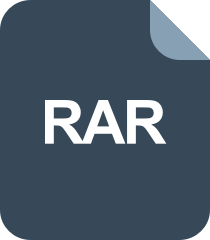
17类花朵数据集.rar

好的,下面是设计的代码:
```cpp
#include <iostream>
#include <sstream>
using namespace std;
class Book {
private:
int id;
string bookTitle;
double price;
string publisher;
static double bookTotalPrice;
public:
Book(int id, string bookTitle, double price, string publisher) {
this->id = id;
this->bookTitle = bookTitle;
this->price = price;
this->publisher = publisher;
bookTotalPrice += price;
}
static double getBookTotalPrice() {
return bookTotalPrice;
}
string getMessage() {
stringstream oss;
oss << "书号:" << id << ", 书名:" << bookTitle << ", 价格:" << price << ", 出版社:" << publisher;
return oss.str();
}
};
double Book::bookTotalPrice = 0;
int main() {
Book book1(1, "C++ Primer", 59.9, "人民邮电出版社");
Book book2(2, "深入浅出设计模式", 49.9, "电子工业出版社");
Book book3(3, "算法导论", 88.8, "机械工业出版社");
cout << book1.getMessage() << endl;
cout << book2.getMessage() << endl;
cout << book3.getMessage() << endl;
cout << "总价:" << Book::getBookTotalPrice() << endl;
return 0;
}
```
这段代码实现了一个图书类Book,其中包括书号(id)、书名(bookTitle)、价格(price)、出版社(publisher)四个数据成员。它还有带4个参数的构造函数,静态数据成员图书对象总价bookTotalPrice,静态函数getBookTotalPrice返回当前图书对象的总价格,以及getMessage()成员函数返回图书信息。
在main函数中,我们构建了三个图书类对象,并测试了类中的所有函数。其中,通过调用getMessage()函数,打印了每本书的信息。最后,通过调用getBookTotalPrice()函数,打印出了所有图书的总价。
阅读全文
相关推荐
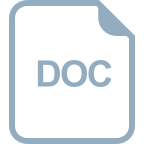
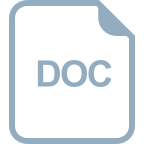















