python实现图像配准
时间: 2023-07-11 15:28:37 浏览: 127
下面是一个使用OpenCV库实现图像配准的示例代码:
```python
import cv2
import numpy as np
# 加载需要配准的两个图像
img1 = cv2.imread('image1.jpg')
img2 = cv2.imread('image2.jpg')
# 提取图像中的SIFT特征点
sift = cv2.xfeatures2d.SIFT_create()
kp1, des1 = sift.detectAndCompute(img1, None)
kp2, des2 = sift.detectAndCompute(img2, None)
# 使用Brute-Force匹配算法匹配特征点
bf = cv2.BFMatcher()
matches = bf.knnMatch(des1, des2, k=2)
# 使用RANSAC算法去除错误的匹配点
good_matches = []
for m, n in matches:
if m.distance < 0.75 * n.distance:
good_matches.append(m)
src_pts = np.float32([kp1[m.queryIdx].pt for m in good_matches]).reshape(-1, 1, 2)
dst_pts = np.float32([kp2[m.trainIdx].pt for m in good_matches]).reshape(-1, 1, 2)
# 计算变换矩阵(采用RANSAC算法去除误匹配点)
M, mask = cv2.findHomography(src_pts, dst_pts, cv2.RANSAC, 5.0)
# 将img1映射到img2上
h, w = img1.shape[:2]
aligned_img = cv2.warpPerspective(img1, M, (w, h))
# 显示配准后的图像
cv2.imshow('Aligned Image', aligned_img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
这个例子使用SIFT特征点检测算法和Brute-Force匹配算法匹配图像中的特征点。然后使用RANSAC算法去除错误匹配点,并计算出变换矩阵将一个图像映射到另一个图像。最后,将一个图像对齐到另一个图像并显示配准后的图像。
相关推荐
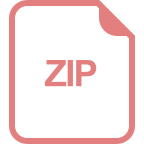
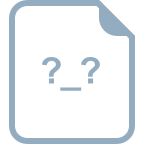
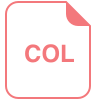
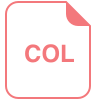
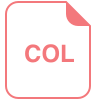
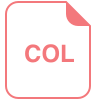
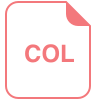









