python代码实现图像配准
时间: 2023-10-13 12:05:09 浏览: 165
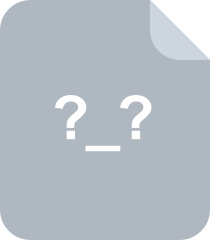
图像配准代码

图像配准是将多个图像对齐使得它们在同一几何坐标系下具有相似的形状、大小和方向。以下是使用Python实现图像配准的步骤和代码示例:
1. 导入所需的库:
```python
import cv2
import numpy as np
```
2. 加载待配准的图像:
```python
image1 = cv2.imread('image1.jpg', 0)
image2 = cv2.imread('image2.jpg', 0)
```
3. 提取关键特征点:
```python
# 创建ORB特征检测器
orb = cv2.ORB_create()
# 在图像中检测关键特征点和描述符
keypoints1, descriptors1 = orb.detectAndCompute(image1, None)
keypoints2, descriptors2 = orb.detectAndCompute(image2, None)
```
4. 使用特征描述符进行匹配:
```python
# 创建BFMatcher对象
bf = cv2.BFMatcher(cv2.NORM_HAMMING, crossCheck=True)
# 使用描述符进行匹配
matches = bf.match(descriptors1, descriptors2)
# 按照匹配程度排序
matches = sorted(matches, key=lambda x: x.distance)
# 选择前n个最佳匹配
n = 50
best_matches = matches[:n]
# 提取关键点位置
points1 = np.float32([keypoints1[m.queryIdx].pt for m in best_matches]).reshape(-1, 1, 2)
points2 = np.float32([keypoints2[m.trainIdx].pt for m in best_matches]).reshape(-1, 1, 2)
```
5. 使用找到的匹配点对进行图像配准:
```python
# 使用RANSAC算法估计单应性矩阵
homography, _ = cv2.findHomography(points1, points2, cv2.RANSAC)
# 对第一个图像进行透视变换
aligned_image = cv2.warpPerspective(image1, homography, (image2.shape[1], image2.shape[0]))
```
完整的代码示例:
```python
import cv2
import numpy as np
# 加载图像
image1 = cv2.imread('image1.jpg', 0)
image2 = cv2.imread('image2.jpg', 0)
# 创建ORB特征检测器
orb = cv2.ORB_create()
# 在图像中检测关键特征点和描述符
keypoints1, descriptors1 = orb.detectAndCompute(image1, None)
keypoints2, descriptors2 = orb.detectAndCompute(image2, None)
# 创建BFMatcher对象
bf = cv2.BFMatcher(cv2.NORM_HAMMING, crossCheck=True)
# 使用描述符进行匹配
matches = bf.match(descriptors1, descriptors2)
# 按照匹配程度排序
matches = sorted(matches, key=lambda x: x.distance)
# 选择前n个最佳匹配
n = 50
best_matches = matches[:n]
# 提取关键点位置
points1 = np.float32([keypoints1[m.queryIdx].pt for m in best_matches]).reshape(-1, 1, 2)
points2 = np.float32([keypoints2[m.trainIdx].pt for m in best_matches]).reshape(-1, 1, 2)
# 使用RANSAC算法估计单应性矩阵
homography, _ = cv2.findHomography(points1, points2, cv2.RANSAC)
# 对第一个图像进行透视变换
aligned_image = cv2.warpPerspective(image1, homography, (image2.shape[1], image2.shape[0]))
# 显示配准后的图像
cv2.imshow('Al
阅读全文
相关推荐


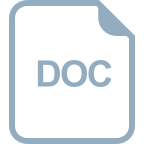











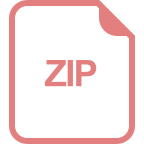
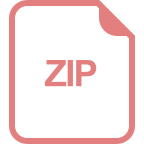