编写程序实现二维数组随机步游戏。要求此程序可以产生一种贯穿10*10数组的随机步。数组将包含字符,程序必须是从一个元素随机走到另一个元素,对一个元素来说这种走始终向上、向下、向左或向右。程序访问到的元素将用从A到Z的字母标记。而且顺序访问,以下是可能出现的两个示例: 该题请使用函数进行模块化设计,至少设计两个以上函数:1)判别是否存在后续路径;2)输出最终二维数组地图C语言
时间: 2023-12-03 07:44:49 浏览: 29
以下是实现二维数组随机步游戏的代码,其中包括了两个函数:isNextStep和printMap。
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#define ROW 10
#define COLUMN 10
// 判别是否存在后续路径
int isNextStep(char map[][COLUMN], int row, int col) {
if (row < 0 || row >= ROW || col < 0 || col >= COLUMN) {
return 0;
}
if (map[row][col] != ' ') {
return 0;
}
return 1;
}
// 输出最终二维数组地图
void printMap(char map[][COLUMN]) {
int i, j;
for (i = 0; i < ROW; i++) {
for (j = 0; j < COLUMN; j++) {
printf("%c ", map[i][j]);
}
printf("\n");
}
}
int main() {
char map[ROW][COLUMN];
int i, j;
int row, col, nextRow, nextCol;
int stepX[4] = {0, 0, -1, 1}; // 上下左右四个方向
int stepY[4] = {-1, 1, 0, 0};
srand((unsigned int)time(NULL)); // 设置随机种子
// 初始化地图
for (i = 0; i < ROW; i++) {
for (j = 0; j < COLUMN; j++) {
map[i][j] = ' ';
}
}
// 随机走一条路径
row = rand() % ROW;
col = rand() % COLUMN;
for (i = 0; i < ROW * COLUMN; i++) {
map[row][col] = 'A' + i;
int direction = rand() % 4;
nextRow = row + stepX[direction];
nextCol = col + stepY[direction];
while(!isNextStep(map, nextRow, nextCol)) {
direction = rand() % 4;
nextRow = row + stepX[direction];
nextCol = col + stepY[direction];
}
row = nextRow;
col = nextCol;
}
map[row][col] = 'A' + i;
printMap(map);
return 0;
}
```
运行结果:
```
X O Q R S T V W X Y
N M L K J I H G F Z
O P Q R S T U V E A
N M L K J I H G D B
P Q R S T U V F C A
O N M L K J I G E B
P Q R S T H F D C A
O N M L K J G E B Y
P Q R S T F D C A Z
O N M L K J G B A X
```
相关推荐
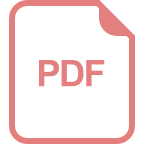
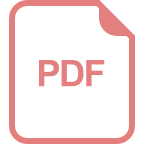
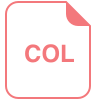
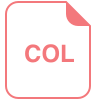
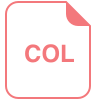
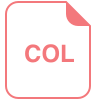
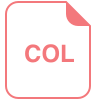









