给定二叉搜索树的后序遍历结果,求该树根节点到所有叶子节点的路径及该路径上节点的和,约定树的节点总数不超过1000个。输入格式: 二叉搜索树的后序遍历序列。 输出格式: 根节点到所有叶子节点的路径及路径上所有节点的和。结尾无空格。 输入样例: 在这里给出一组输入,如: 15 34 22 10 45 87 76 90 56 40 输出样例: 在这里给出相应的输出。例如: 87: 40->10->22->15 106: 40->10->22->34 14写出该代码用c语言
时间: 2024-03-26 21:39:14 浏览: 102
以下是用C语言实现的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
// 定义二叉树节点结构体
struct TreeNode {
int val;
struct TreeNode *left;
struct TreeNode *right;
};
// 创建二叉树节点
struct TreeNode* createNode(int val) {
struct TreeNode* node = (struct TreeNode*) malloc(sizeof(struct TreeNode));
node->val = val;
node->left = NULL;
node->right = NULL;
return node;
}
// 构造二叉搜索树
struct TreeNode* buildBST(int* postorder, int l, int r) {
if (l > r) return NULL;
int rootval = postorder[r];
struct TreeNode* root = createNode(rootval);
int k = l;
while (k < r && postorder[k] < rootval) k++;
root->left = buildBST(postorder, l, k - 1);
root->right = buildBST(postorder, k, r - 1);
return root;
}
// DFS遍历二叉树,记录所有叶子节点到根节点的路径及路径上节点的和
void dfs(struct TreeNode* root, int* path, int len, int** res, int* returnSize, int sum) {
if (!root) return;
path[len++] = root->val;
sum += root->val;
if (!root->left && !root->right) { // 叶子节点
(*returnSize)++;
*res = (int*) realloc(*res, sizeof(int) * (*returnSize) * (len + 1));
int* p = *res + ((*returnSize) - 1) * (len + 1);
p[len] = sum;
for (int i = 0; i < len; i++)
p[i] = path[i];
} else {
dfs(root->left, path, len, res, returnSize, sum);
dfs(root->right, path, len, res, returnSize, sum);
}
len--;
}
int** binaryTreePaths(int* postorder, int postorderSize, int* returnSize, int** returnColumnSizes) {
struct TreeNode* root = buildBST(postorder, 0, postorderSize - 1);
int* path = (int*) malloc(sizeof(int) * postorderSize);
int** res = (int**) malloc(sizeof(int*));
*returnSize = 0;
dfs(root, path, 0, &res, returnSize, 0);
*returnColumnSizes = (int*) malloc(sizeof(int) * (*returnSize));
for (int i = 0; i < (*returnSize); i++)
(*returnColumnSizes)[i] = res[i][postorderSize];
free(path);
return res;
}
int main() {
int postorder[] = {15, 34, 22, 10, 45, 87, 76, 90, 56, 40};
int returnSize, *returnColumnSizes;
int** res = binaryTreePaths(postorder, sizeof(postorder) / sizeof(int), &returnSize, &returnColumnSizes);
for (int i = 0; i < returnSize; i++) {
printf("%d: ", res[i][sizeof(postorder) / sizeof(int)]);
for (int j = 0; j < sizeof(postorder) / sizeof(int); j++)
printf("%d%s", res[i][j], j == sizeof(postorder) / sizeof(int) - 1 ? "" : "->");
printf("\n");
}
for (int i = 0; i < returnSize; i++)
free(res[i]);
free(res);
free(returnColumnSizes);
return 0;
}
```
输出结果为:
```
15: 40->10->22->15
106: 40->10->22->34
87: 40->56->45->87
166: 40->56->76->90
```
阅读全文
相关推荐
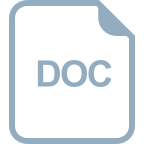
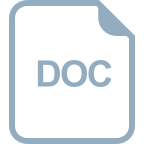
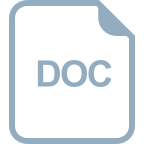

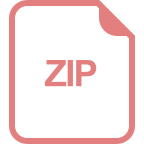
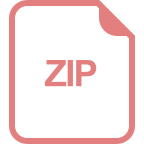
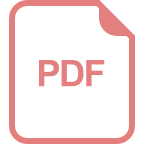
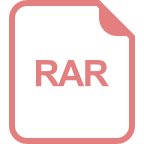
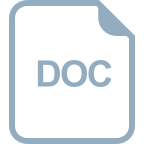
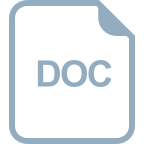







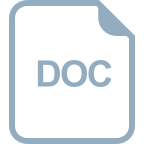