ValueError: could not convert string '8,8,0,4.2504e+005,0.065,-0.058' to float64 at row 0, column 1.
时间: 2024-10-19 14:02:17 浏览: 34
这个错误提示是在Python中发生的,通常当你尝试将字符串转换为浮点数(`float64`),但字符串的内容无法被成功解析为有效的数字时会出现这种错误。在这个例子中,字符串`'8,8,0,4.2504e+005,0.065,-0.058'`在试图转换时遇到了问题,可能是由于逗号分隔的数值中有非数字字符,或者科学记数法(`e+005`)表示的不是有效数字。
解决这个问题需要检查字符串内容,确保所有的部分都是可以转换成数字的。例如,你可以通过先删除逗号或其他非数字字符,然后使用`try-except`结构来捕获并处理转换异常。如果字符串包含科学记数法,确认它格式正确并且能够被`float`函数接受。
```python
data = '8,8,0,4.2504e+005,0.065,-0.058'
numbers = [float(num.strip(',').replace('e', 'e+')) for num in data.split(',')]
# 如果有异常发生,这里会捕获并处理
try:
numbers = [float(num) for num in data.split(',')]
except ValueError as e:
print(f"Error converting {num}: {str(e)}")
```
相关问题
ValueError: could not convert string to float: '0.953952 largeDoses'
这个错误提示表明在尝试将字符串转换为浮点数时出现了问题。具体来说,程序试图将字符串 '0.953952 largeDoses' 转换为浮点数,但无法做到这一点,因为字符串中包含了非数字字符。
解决这个问题的方法取决于你的具体需求。如果你只是想将该字符串视为一个字符串,那么可以忽略这个错误。但如果你需要将该字符串转换为浮点数,你需要确保该字符串只包含数字字符。
以下是一些可能的解决方案:
1. 检查输入数据:确保你正在尝试转换的数据确实只包含数字字符。
2. 使用正则表达式:如果你不确定输入数据是否只包含数字字符,可以使用正则表达式来验证。例如,你可以使用Python的re模块来检查字符串是否只包含数字:
```python
import re
input_str = '0.953952 largeDoses'
if re.fullmatch(r'^\d+(\.\d+)?$', input_str):
# 转换成功,继续处理
float_value = float(input_str)
else:
# 转换失败,处理错误情况
print("无法将字符串转换为浮点数")
```
这段代码使用正则表达式来检查输入字符串是否只包含数字和可能的点号。如果字符串符合这个模式,那么它将被成功转换为浮点数。否则,将打印出错误消息。
请注意,这只是一种可能的解决方案,具体的解决方案可能取决于你的具体需求和数据情况。
ValueError: could not convert string to float: 'admin.'
This error is likely occurring because you are trying to convert the string 'admin.' to a float using a method such as float('admin.'). This is not possible because 'admin.' is not a valid float value.
To resolve this error, you need to ensure that you are only trying to convert valid float values to floats. Check the data you are working with to make sure that all values that you are attempting to convert to floats are actually numeric values. If there are non-numeric values mixed in with your data, you will need to clean or filter the data before attempting to convert it to floats.
阅读全文
相关推荐















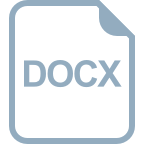
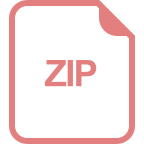