java实现模拟退火算法
时间: 2024-04-14 18:24:45 浏览: 14
Java可以通过编写代码来实现模拟退火算法。模拟退火算法是一种全局优化算法,常用于解决复杂的优化问题。下面是一个简单的Java实现模拟退火算法的示例:
```java
import java.util.Random;
public class SimulatedAnnealing {
public static void main(String[] args) {
// 初始化参数
double initialTemperature = 100; // 初始温度
double coolingRate = 0.95; // 降温速率
int numIterations = 1000; // 迭代次数
// 初始化当前解和最优解
double currentSolution = generateRandomSolution();
double bestSolution = currentSolution;
// 开始模拟退火算法
for (int i = 0; i < numIterations; i++) {
// 生成新解
double newSolution = generateRandomSolution();
// 计算能量差
double energyDifference = newSolution - currentSolution;
// 判断是否接受新解
if (energyDifference < 0 || Math.exp(-energyDifference / initialTemperature) > Math.random()) {
currentSolution = newSolution;
}
// 更新最优解
if (currentSolution < bestSolution) {
bestSolution = currentSolution;
}
// 降温
initialTemperature *= coolingRate;
}
System.out.println("最优解:" + bestSolution);
}
// 生成随机解
private static double generateRandomSolution() {
Random random = new Random();
return random.nextDouble() * 100; // 假设解的范围是0到100
}
}
```
上述代码中,模拟退火算法的主要步骤如下:
1. 初始化参数,包括初始温度、降温速率和迭代次数。
2. 初始化当前解和最优解,将当前解设为一个随机生成的初始解。
3. 开始迭代,每次迭代生成一个新解,并计算能量差。
4. 判断是否接受新解,如果能量差小于0或者满足一定概率条件,则接受新解。
5. 更新最优解。
6. 降温,通过乘以降温速率来降低温度。
7. 重复步骤3到步骤6,直到达到迭代次数。
这是一个简单的模拟退火算法实现示例,你可以根据具体问题进行适当的修改和扩展。
相关推荐
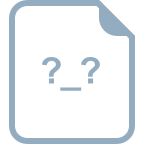
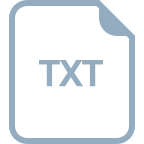
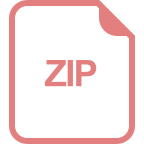














